Eton: an Overview of the Elegant eCommerce Store
Find out how to make your store looking good, and give your customers the best shopping experience with custom features used throughout the whole buyer journey.
The story of this brand begins with one family, kitchen, and dresses. Annie Petterson sewed dresses, and David Petterson worked in a sawmill – a small family business. When The Great Depression hit in 1920, and David’s business had to close, David and his wife Annie joined their talents and efforts to create their small company named Specialized Sewing Factory. The business was growing, their living area was known for textile manufacturing, and it was easy to expand. However, choosing the right product helped to develop such a strong shirt brand.
Next few years, the family business became even more recognized when Annie and David’s sons – Rune and Arne Davidson were traveling around the world in search of new fabrics. The inspiration and new materials were taken from a small town named Eton.
That’s how the brand Eton was expanded to England and got its original name. The history of the brand was highlighted with success and innovations that helped them to stay famous and competitive on the market. After 90 years of work, Swedish brand Eton has grown from a small family business started after the failure to a luxury fashion house with flagship stores in London, New York, Frankfurt, and Stockholm.
But what exactly makes Eton different among other sewing companies?
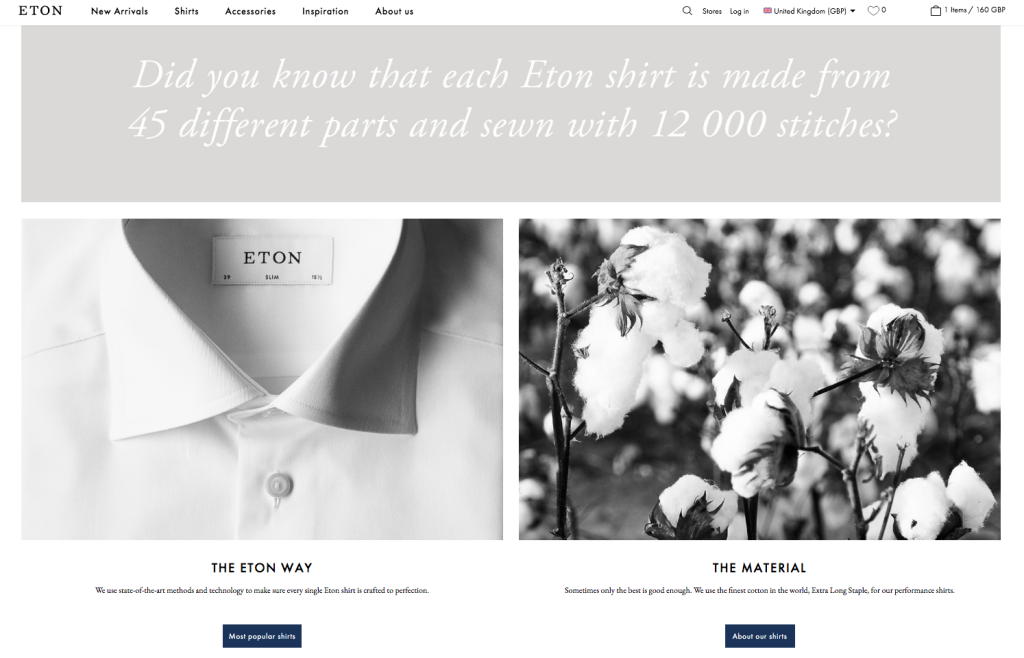
The first reason is experience. Shirts have been crafted for more than 90 years, and have the best materials and techniques used for their production. The company claims to be sustainable, so they are experimenting with Tencel. However, the most famous fiber in production is cotton. Eton uses the best quality of cotton, and some particular types of it to produce their shirts.
Eton also works on optimizing all the production processes, including shirts’ design. The brand collaborates with young artists to create unique printing designs and offers a classic style of shirts like white or blue. These defining features make Eton a famous luxury shirt brand.
However, our first impression was from their online store. It exactly corresponds to the brand’s vision and translates all the values and benefits of it. We’ve checked their site to provide you with a review of their awesome features.
Here is the list of insights that you can use for your brand’s growth and which we’ll look into in this article:
- Tell your brand’s story. The best place to present your brand’s values, mission, and build strong relationships with your customers is your website. You can do it by creating the About Us page, highlighting your key advantages, and other marketing pages to gain customers’ loyalty.
- Lead your customers to the purchase. You can use banners on the homepage to show your best products or deals, or create specific categories to help customers choose products for special occasions. Also, additional guides on how to use your product or brand-related stories will help customers choose the desired product faster, which leads to more sales.
- Organize a comfortable shopping experience. Convenience and clear navigation are what a customer wants from the store. That is why a detailed description of the product, additional blocks for various product types, sizes, and related products will help you to sell more products. Adding reviews, care guide, and informational pages as FAQ, Delivery, and Returns pages will help to clarify all the working processes for customers and makes them feel confident about their choice.
- Add some entertaining details. Eton has beautiful and informative pages for clients to make them want to purchase their product (we’ll see it below). This can be some inspiration on how to dress, style, or use your product, the story of your brand or featured products, product details with a description, and another kind of informational pages. Anything clickable or moving and animated will be interesting to look at. You can add video or animation to the pages, and blocks that are hidden in the product images or banners.
Let’s continue with an overview of the website and see HOW and WHY Eton’s website features can be useful for your business. You can use these insights to improve the user experience at your store and increase your sales.
Website Overview
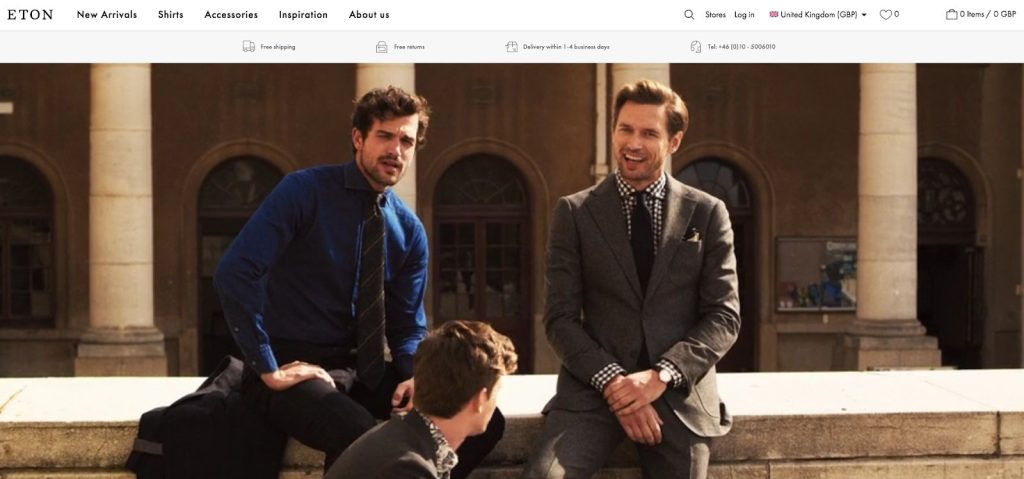
When you get to the main page, first you see the video with 3 men wearing shirts from the latest collection. The whole website is telling a story about the brand, the quality of their shirts, transparency of business processes, and make you feel like a part of a luxury men’s club. Colors of the website are calm, and at the same time, the design is transferring the confidence of this brand.
If we will look at the brand, and how it translates their vision of the product through the website, we’ll see that a product is not just a plain shirt. It is a part of the look, a unique experience with long-lasting quality, and for special occasions. With this in mind, we’ll check the most interesting features and pages that the Eton team has created for their customers, providing them with the best user experience.
Now, let’s consider each part of the website in detail.
Top Useful Features of the Store
Eton store has convenient navigation with lots of categories for different occasions. Let’s review the desktop version of the store.
Key Benefits on the Main Page to Catch Attention & Show Value
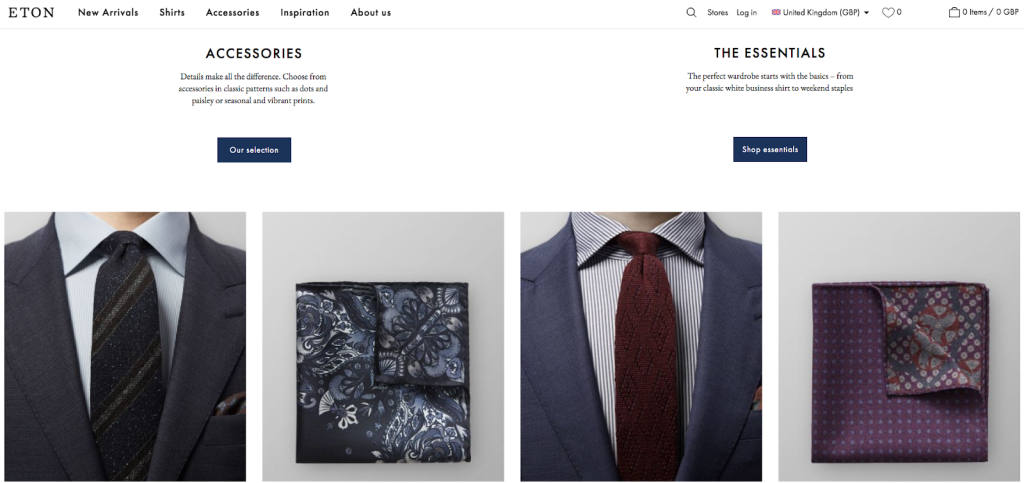
The sections with various categories on the main page are showcasing all the important collections and products. This is great because any person who visits the store can easily buy any product. The header also has a selection of currencies, customer account, cart, and favorites, which is convenient for a customer to browse and buy freely.
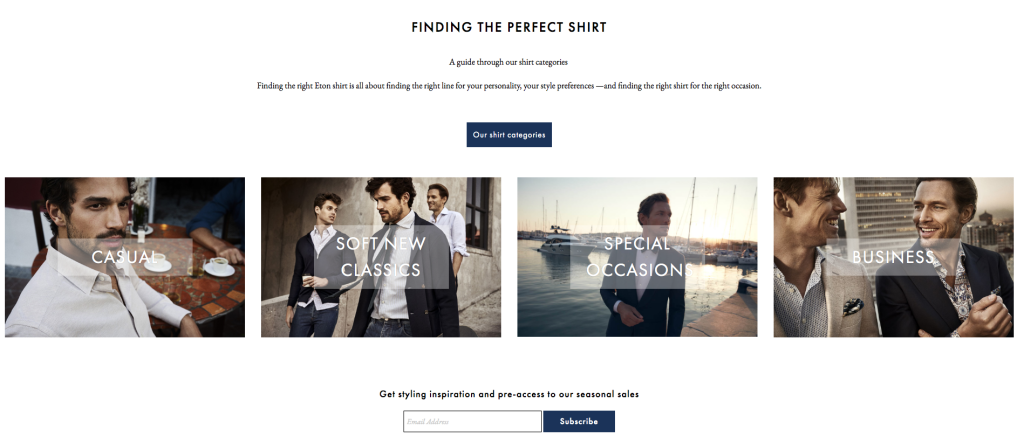
High-Quality Resized Images to Showcase the Products
A customer gets the resized image of the print. It is very convenient, as the size of the image tends to be small, especially on mobile. In this case, you can reach more customers with a clear view of each product they buy. Also, this helps to showcase the quality of each fabric and to prove the quality of the shirts.
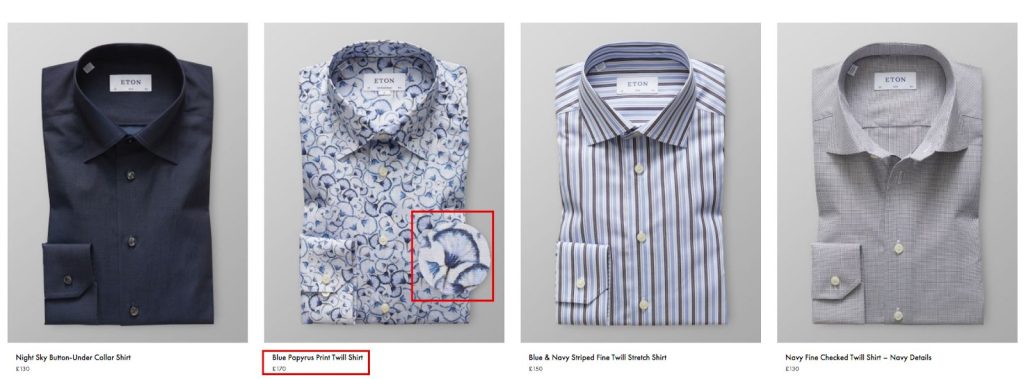
Categories for Various Occasions to Increase Sales
As we’ve already mentioned, Eton has created various categories to help its customers find the right product. It’s a great idea because people tend to choose clothes for the occasion, and they will likely buy several products if they go well with each other.
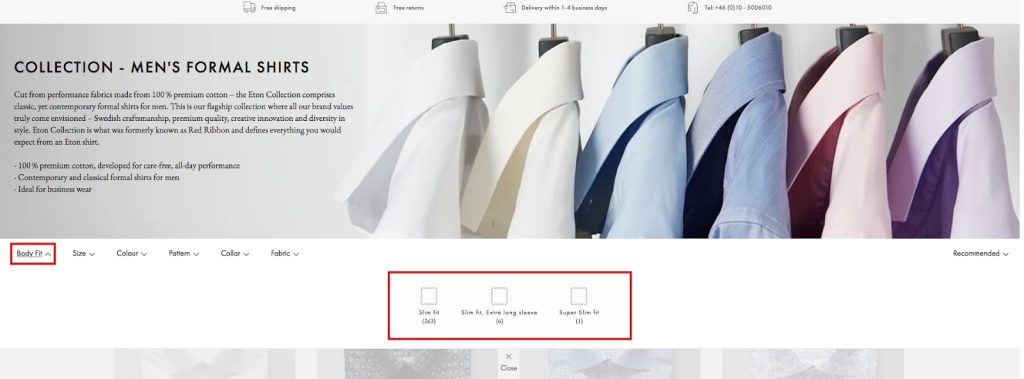
Product filters show all the different styles, patterns, fabrics, and collars, so a customer sees the whole range of the products. It is helpful for customers in terms of narrowing down the choices. With these filters, they are able to choose the exact product they want.
Care Guide to Reduce Customers Anxiety
The product page has lots of unique features made for customers. For example, you have a care guide which opens in a pop-up and has a detailed description of how to take care of the shirt. This helps to learn all the information regarding the product, and avoid unawareness regarding the quality after washing it.
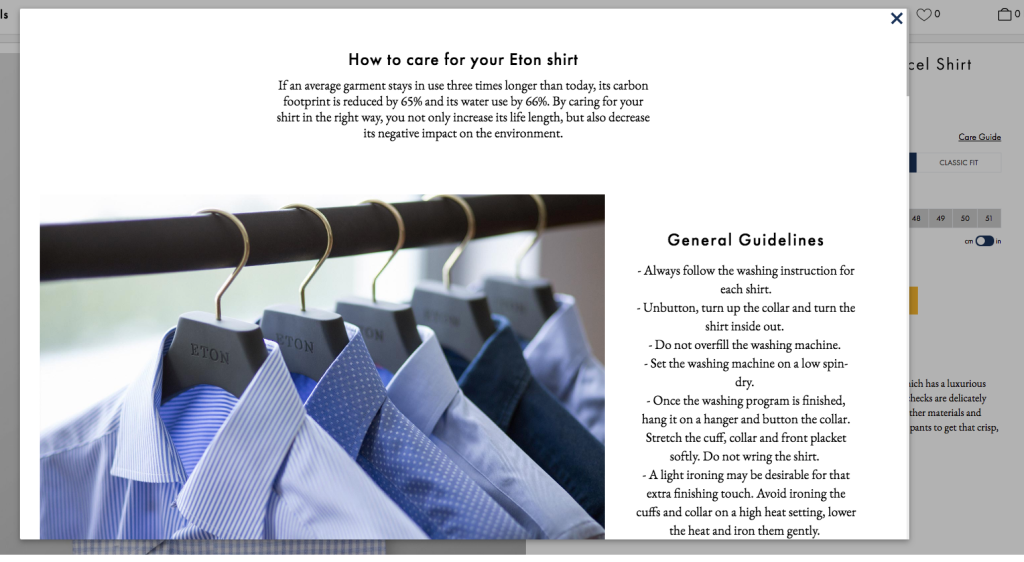
Also, a customer can choose one of the fit types for your preference: slim or classic, or another.
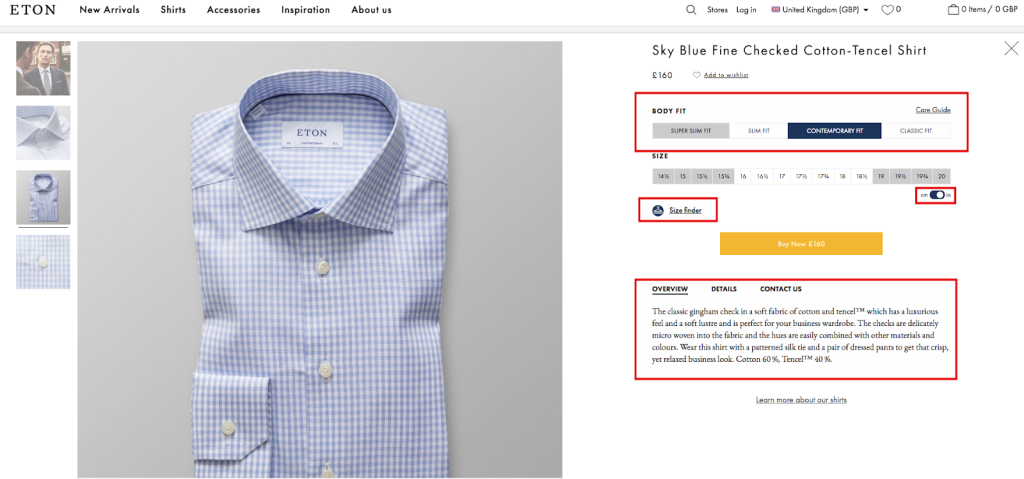
User-Friendly Product Card to Motivate Purchase
The overview and details of the product are written in short with the most important information regarding the material, style, and product care. The page includes fit finder, which helps to define the size of the product based on your data. This feature is helpful for those customers who tend to get worried about the wrong size and possible returns, and overall add more engagement in the buying process.
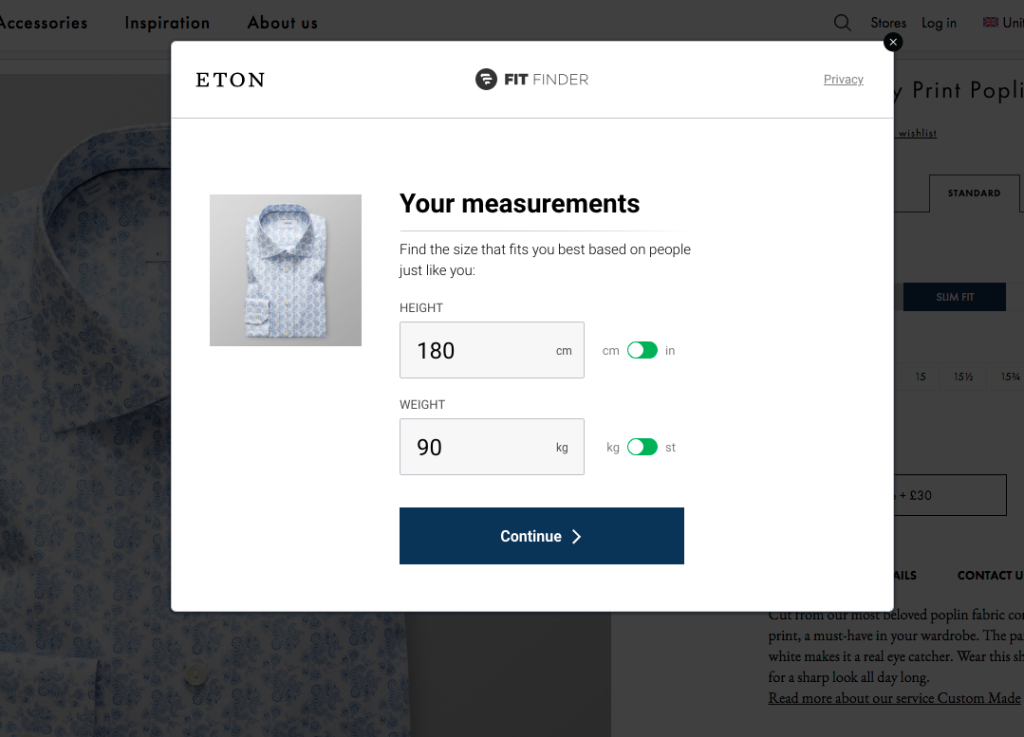
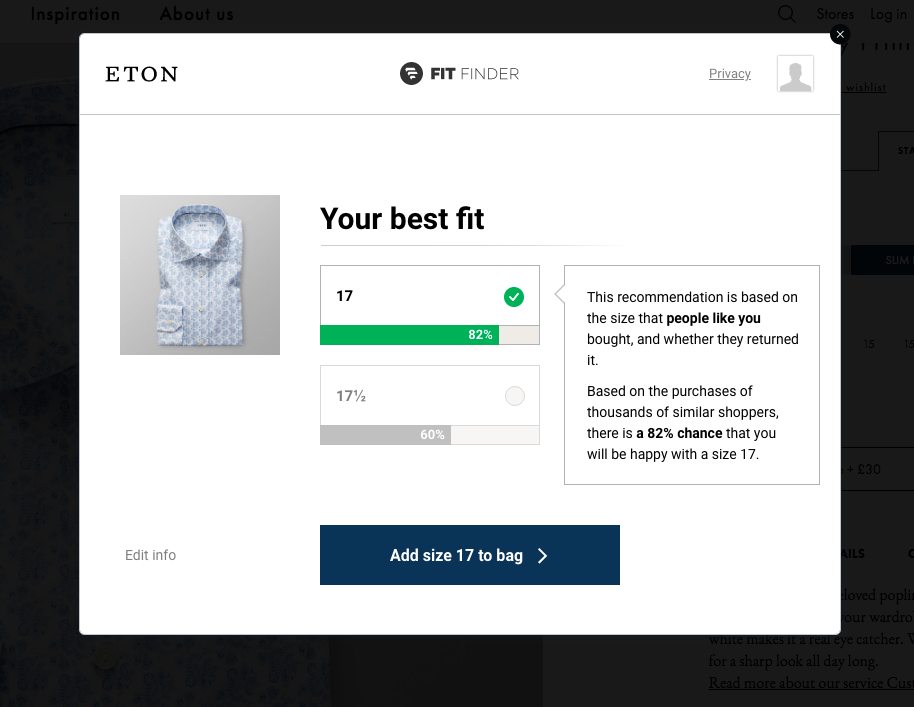
Detailed Size Guide to Keep Customer Entertained
If a customer wants to know how to find the right fit for the specific shirt, Eton has the page called Size Guide with all the sizes, and instructions on how to choose the right one.
The next unique feature that Eton turned into their unique proposition is a customization of the shirt on the product page. A person can select what to change in the basic option, and it will cost £30. This includes the collar, cuffs, and front. They can also add a monogram, change the length of the shirt and sleeves. The benefit for customers is a custom-designed shirt which makes them feel special and unique. Plus a custom made products cost more, and customers tend to pay more for the additional services without any doubts.
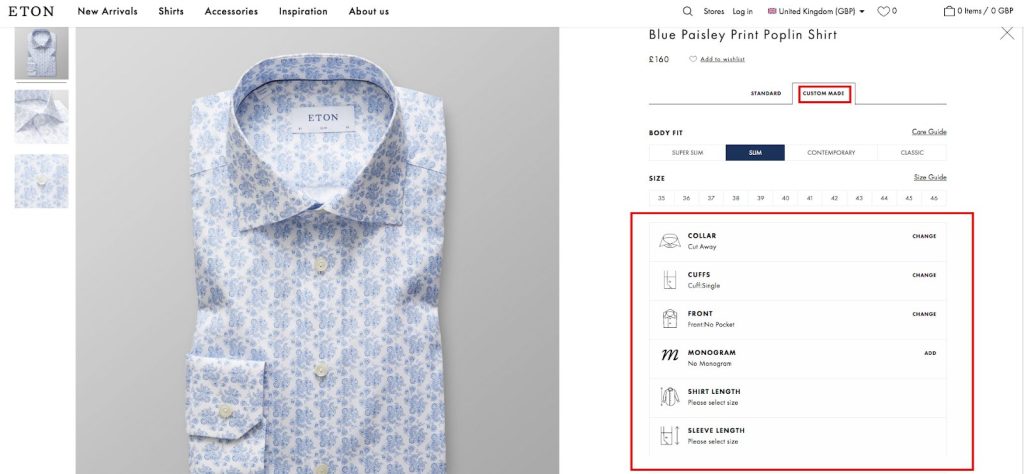
Simple Checkout Process for Smooth and Impulse Purchases
Eton has one page to fill out the customer’s information. This is much more convenient than having 2-3 pages; however, it requires some time to fill out the data which could cause difficulty for some customers.
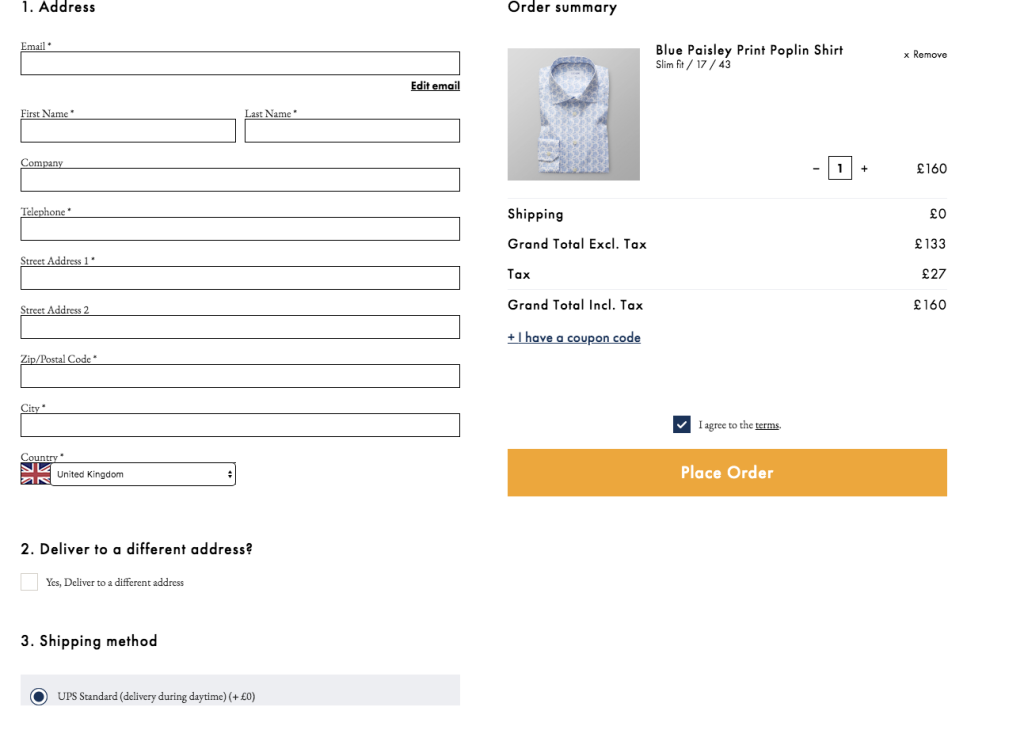
According to Neil Patel, the abandonment rate happens at the first step of checkout because of the “sign-in” barrier. If you’ll simplify the checkout process, place fields in the right order (first should be the address than shipping, and last is billing not to frustrate customers with the payment right away). If your checkout is done right, the conversion rate can increase up to 5%.
Great Marketing Pages to Convince the Customers
Next part was the most pleasant to review. This includes Inspiration, Sustainability, and About Us page. They bring the value of the brand, reduce anxieties, and let customers having a desire to get their products.
Other pages are helpful in terms of customer care and service. They give information about the product, entertain, and interact with a customer directly. The information pages of Eton site such as “About Us” and “Care Guide” created for customers with care. They cover all the questions that might occur regarding the product, manufacturing, and other customer-related answers.
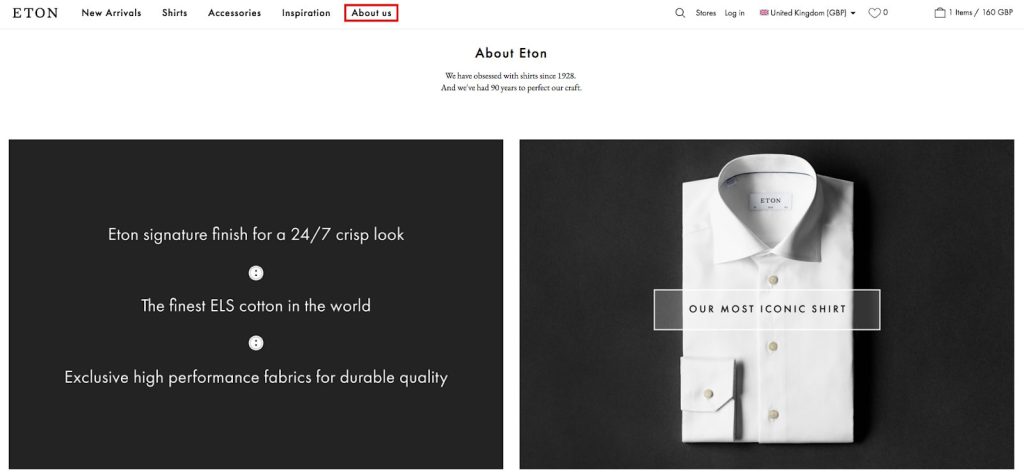
Pages look great and give the customer a good feeling about Eton products even before they purchase anything.
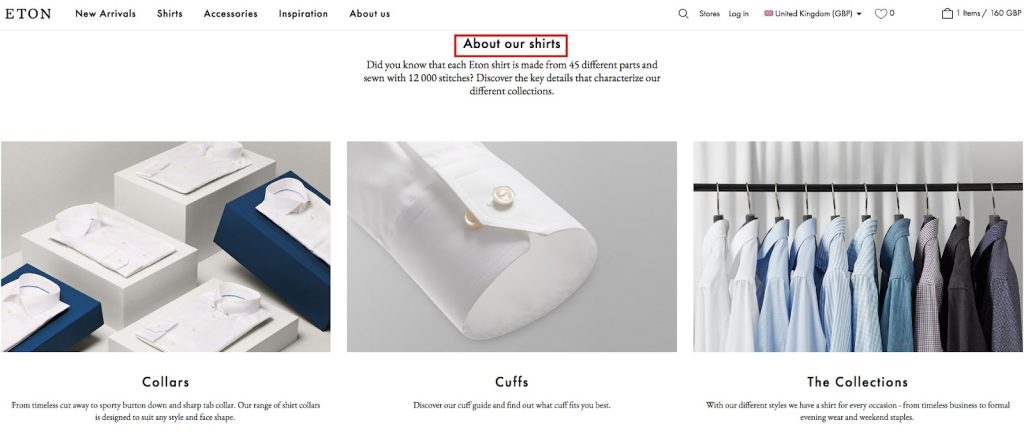
Informational Pages to Show Brand Purpose and Impact
Informational pages, such as Sustainability, cover questions of responsibility of the company in the manufacturing and delivery of the products.
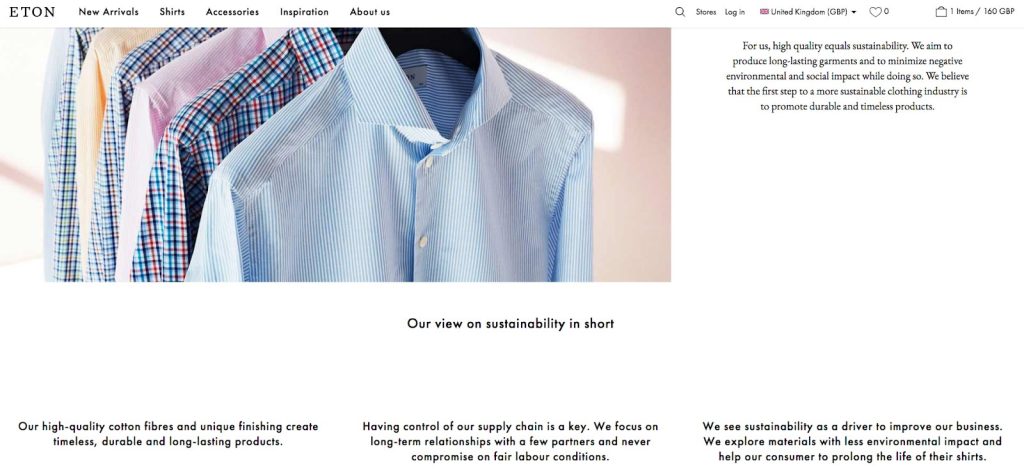
They also provide Sustainability reports, and Code of Conduct, which means that Eton is a part of certified companies in a list of world-leading sustainable organizations. Any eligible or international certificates and awards will show the expertise and the quality of products/services of the company. That’s why it is better to highlight the awards of your company in the footer.
Useful for you:
Instead of the Conclusion
As you can see, Eton e-store is an excellent example of a user-friendly website, and the brand itself is a real hero of a fantastic success story. We were impressed with how Eton founders have overcome all failures and barriers rising their new successful star, which is now one of the TOP Swedish fashion brands. You see that everything is possible if you are committed to your idea and ready to be flexible in your strategy.
We’ve made an overview of Eton store features. It was a pleasure because this store for men has a protruding portion of aesthetics and care which couldn’t leave customers unconcerned.
We know how important it is to keep customers satisfied. That’s why we encourage you to use various features to make your site look great and develop your brand to be outstanding. Try UX tips from this guide, and you’ll see how your sales increase.
Magento 2.2 Bug Preventing Users from Updating Their Passwords, and a Method for Fixing It
There is a bug in Magento 2.2, starting with version 2.2.6, which prevents users from resetting their passwords if they forget them. When attempting to reset their passwords, users get an error message saying, “Something went wrong while saving the new password.”
The reason for this error was specified here: https://github.com/magento/magento2/issues/18256. The error was resolved in version 2.3, and it involved the sequence of operators responsible for clearing the session of the resetPassword method of the app/code/Magento/Customer/Model/AccountManagement.php class. People who are still using version 2.2.6 or 2.2.7 can install a patch, but there’s another way to fix this problem. You can fix the bug in the third-party module and in so doing avoid this error. We will examine how to do this.
Eliminating the Bug in the Third-Party Module
To eliminate the bug, we have to replace the app/code/Magento/Customer/Model/AccountManagement.php class in the Magento\Customer\Controller\Account\ResetPasswordPost controller, using a dependence-injection like this:
<type name="Magento\Customer\Controller\Account\ResetPasswordPost">
<arguments>
<argument name="accountManagement"
xsi:type="object">Web4pro\Wholesale\Model\AccountManagement</argument>
</arguments>
</type>
It’s not advised to redefine through preference, but since we only need to fix the problem within the limits of one controller call, this method will work. Another difficulty is that the majority of the methods and properties of the original class are private. Because of this, a given class will look like this:
class AccountManagement extends \Magento\Customer\Model\AccountManagement
{
protected $customerRepository;
protected $credentialsValidator;
protected $customerRegistry;
protected $sessionManager;
protected $searchCriteriaBuilder;
protected $scopeConfig;
protected $dateTimeFactory;
protected $visitorCollectionFactory;
protected $saveHandler;
public function __construct(CustomerFactory $customerFactory, ManagerInterface $eventManager,
StoreManagerInterface $storeManager, Random $mathRandom, Validator $validator,
ValidationResultsInterfaceFactory $validationResultsDataFactory, AddressRepositoryInterface
$addressRepository, CustomerMetadataInterface $customerMetadataService, CustomerRegistry
$customerRegistry, PsrLogger $logger, Encryptor $encryptor, ConfigShare $configShare, StringHelper
$stringHelper, CustomerRepositoryInterface $customerRepository, ScopeConfigInterface $scopeConfig,
TransportBuilder $transportBuilder, DataObjectProcessor $dataProcessor, Registry $registry, CustomerViewHelper
$customerViewHelper, DateTime $dateTime, CustomerModel $customerModel, ObjectFactory $objectFactory,
ExtensibleDataObjectConverter $extensibleDataObjectConverter, CredentialsValidator $credentialsValidator =
null, DateTimeFactory $dateTimeFactory = null, AccountConfirmation $accountConfirmation = null,
SessionManagerInterface $sessionManager = null, SaveHandlerInterface $saveHandler = null, CollectionFactory
$visitorCollectionFactory = null, SearchCriteriaBuilder $searchCriteriaBuilder = null)
{
$this->customerRepository = $customerRepository;
$this->customerRegistry = $customerRegistry;
$this->sessionManager = $sessionManager;
$this->searchCriteriaBuilder = $searchCriteriaBuilder?:ObjectManager::getInstance()-
>get(SearchCriteriaBuilder::class);
$this->credentialsValidator = $credentialsValidator?: ObjectManager::getInstance()-
>get(CredentialsValidator::class);
$this->scopeConfig = $scopeConfig;
$this->dateTimeFactory = $dateTimeFactory ?: ObjectManager::getInstance()-
>get(DateTimeFactory::class);
$this->visitorCollectionFactory = $visitorCollectionFactory?:ObjectManager::getInstance()-
>get(CollectionFactory::class);
$this->saveHandler = $saveHandler?: ObjectManager::getInstance()-
>get(SaveHandlerInterface::class);
parent::__construct($customerFactory, $eventManager, $storeManager, $mathRandom, $validator,
$validationResultsDataFactory, $addressRepository, $customerMetadataService, $customerRegistry, $logger,
$encryptor, $configShare, $stringHelper, $customerRepository, $scopeConfig, $transportBuilder, $dataProcessor,
$registry, $customerViewHelper, $dateTime, $customerModel, $objectFactory, $extensibleDataObjectConverter,
$credentialsValidator, $dateTimeFactory, $accountConfirmation, $sessionManager, $saveHandler,
$visitorCollectionFactory, $searchCriteriaBuilder);
}
public function resetPassword($email, $resetToken, $newPassword)
{
if (!$email) {
$customer = $this->matchCustomerByRpToken($resetToken);
$email = $customer->getEmail();
} else {
$customer = $this->customerRepository->get($email);
}
//Validate Token and new password strength
$this->validateResetPasswordToken($customer->getId(), $resetToken);
$this->credentialsValidator->checkPasswordDifferentFromEmail(
$email,
$newPassword
);
$this->checkPasswordStrength($newPassword);
//Update secure data
$customerSecure = $this->customerRegistry->retrieveSecureData($customer->getId());
$customerSecure->setRpToken(null);
$customerSecure->setRpTokenCreatedAt(null);
$customerSecure->setPasswordHash($this->createPasswordHash($newPassword));
$this->getAuthentication()->unlock($customer->getId());
$this->destroyCustomerSessions($customer->getId());
$this->sessionManager->destroy();
$this->customerRepository->save($customer);
return true;
}
protected function matchCustomerByRpToken($rpToken)
{
$this->searchCriteriaBuilder->addFilter(
'rp_token',
$rpToken
);
$this->searchCriteriaBuilder->setPageSize(1);
$found = $this->customerRepository->getList(
$this->searchCriteriaBuilder->create()
);
if ($found->getTotalCount() > 1) {
//Failed to generated unique RP token
throw new ExpiredException(
new Phrase('Reset password token expired.')
);
}
if ($found->getTotalCount() === 0) {
//Customer with such token not found.
throw NoSuchEntityException::singleField(
'rp_token',
$rpToken
);
}
//Unique customer found.
return $found->getItems()[0];
}
protected function validateResetPasswordToken($customerId, $resetPasswordLinkToken)
{
if (empty($customerId) || $customerId < 0) { //Looking for the customer. $customerId = $this->matchCustomerByRpToken($resetPasswordLinkToken)
->getId();
}
if (!is_string($resetPasswordLinkToken) || empty($resetPasswordLinkToken)) {
$params = ['fieldName' => 'resetPasswordLinkToken'];
throw new InputException(__('%fieldName is a required field.', $params));
}
$customerSecureData = $this->customerRegistry->retrieveSecureData($customerId);
$rpToken = $customerSecureData->getRpToken();
$rpTokenCreatedAt = $customerSecureData->getRpTokenCreatedAt();
if (!Security::compareStrings($rpToken, $resetPasswordLinkToken)) {
throw new InputMismatchException(__('Reset password token mismatch.'));
} elseif ($this->isResetPasswordLinkTokenExpired($rpToken, $rpTokenCreatedAt)) {
throw new ExpiredException(__('Reset password token expired.'));
}
return true;
}
/**
* Get authentication
*
* @return AuthenticationInterface
*/
protected function getAuthentication()
{
if (!($this->authentication instanceof \Magento\Customer\Model\AuthenticationInterface)) {
return \Magento\Framework\App\ObjectManager::getInstance()->get(
\Magento\Customer\Model\AuthenticationInterface::class
);
} else {
return $this->authentication;
}
}
protected function destroyCustomerSessions($customerId)
{
$sessionLifetime = $this->scopeConfig->getValue(
\Magento\Framework\Session\Config::XML_PATH_COOKIE_LIFETIME,
\Magento\Store\Model\ScopeInterface::SCOPE_STORE
);
$dateTime = $this->dateTimeFactory->create();
$activeSessionsTime = $dateTime->setTimestamp($dateTime->getTimestamp() - $sessionLifetime)
->format(DateTime::DATETIME_PHP_FORMAT);
/** @var \Magento\Customer\Model\ResourceModel\Visitor\Collection $visitorCollection */
$visitorCollection = $this->visitorCollectionFactory->create();
$visitorCollection->addFieldToFilter('customer_id', $customerId);
$visitorCollection->addFieldToFilter('last_visit_at', ['from' => $activeSessionsTime]);
$visitorCollection->addFieldToFilter('session_id', ['neq' => $this->sessionManager->getSessionId()]);
/** @var \Magento\Customer\Model\Visitor $visitor */
foreach ($visitorCollection->getItems() as $visitor) {
$sessionId = $visitor->getSessionId();
$this->saveHandler->destroy($sessionId);
}
}
How to Find Trending Products to Sell Online in 2019
If you’re looking to start an eCommerce business and to develop a solid brand, it is essential that you find the right products for selling. For this reason, it may be important to note which products are trending because this can provide you with a direction to take regarding your business.
We will provide you with a guide on where and how to search for trending products to start your online business in 2019.
How to Find Trending Products to Sell Online?
The best way to start your research lies in search engines and popular marketplaces. They provide you with the most relevant answers and give you plenty of choices to find trending products.
Google is known for providing entrepreneurs and business owners with tools to help them gain success.
Think with Google project provides you with the latest consumer behavior insights, various tools to optimize your current and future website, and useful articles to learn more about the market. Google trends products can also provide you with websites that can introduce you to new products that will help you to build your business.
Google Trends Tool
Google trends tool is one of the best places to find the information you need about trending topics. If you’re looking to work in a specific niche, you can enter keywords that are related to your niche and can find trending products that way.
Amazon
This website, which started as an online bookstore, is another great option for those who are looking to find new and trending products to sell in online stores. When you click on a trending item, a list pops up under the item that shows others you might be interested in.
This is a great way to ensure that you’re always being introduced to new topics that will keep your interest and the interest of potential customers.
AliExpress
AliExpress is another great website to use if you’re looking for new and trending products to sell. This is because you can look at the websites’ Recommended and Top Rated sections to find out what people are most interested in.
Useful for you:
How to Verify Trending Products to Sell Online
After finding some of the top trending products out there, it’s essential that you know how to verify them.
Use Product Sorting and Filtering Apps
Sorting and filtering apps ensure that your eCommerce business can receive insights regarding the most profitable products out there.
AMZScout
This extension and tool allow you to find the best products to sell. With it, you can see growth patterns, and you can decide whether the products shown fit in with your niche.
You can pay for this extension every month. The price is around $45 per month, or you can pay $199 for a lifetime membership.
Unicorn Smasher
This tool provides you with a way to determine which opportunities are best for your individual needs. You can, for example, be provided with an opportunity score that lets you know how different favorable products are.
AmazeOwl
This tool claims to help individuals to launch products on Amazon that will be efficient in both functions and sales. This is the right tool for you if you’re looking for something that can provide you with only the best insights into the world of product sales. It has 3 packages that you can pay monthly or annually. The basic one is free, next is $14,95 per/month, and the Established is $29.95 per/month.
Subscribe to Trendy Publications
Using trendy publications ensures that you’re entirely up to date with the newest information in the field of eCommerce and product sales.
PSFK
This tool provides customers and eCommerce owners with insights into trends, immersive events, and much more.
Trend Hunter
This tool is known for its ability to provide users with real-time data about potential products. If you’re trying to find something that matches your niche or if you’re trying to break into a niche altogether, this is the right one for you.
Cool Hunting
Cool Hunting provides users with insight into new trends with real-time data that ensures your eCommerce business can kick-off to the right start.
Springwise
Springwise specializes in providing customers and eCommerce business owners with information about only the newest innovations and newest trends. This ensures you’re always up to date with what’s going on in your niche.
Check Out Product Review Blogs
Things like product review blogs are some of the most useful tools that you have at your disposal. This is because these blogs will provide you with in-depth insight into the workings and the overall quality of different products.
With the tool above, you’ll be able to determine whether you want to take on this product and whether it’s the right one for your needs.
Scroll Through Social Image Networks
This is one of the best ways for you to find out what people are saying about the product you’re interested in buying and selling.
This social media platform is one of the best ways for you to find innovative projects to work on. Pinterest is known for introducing unique products and ideas that any eCommerce business owner will appreciate.
Fancy
Fancy can provide visitors and buyers with many unique products that can’t be found elsewhere. If you use some of the aforementioned tools to determine which products are trending, using Fancy will allow you to find some of the best and most unique products out there.
Wanelo
Wanelo claims to be one of the best ways for you to shop from your phone. With the help of this application, you can browse through dozens of products to find the ones that are right for you and for your individual niche.
Instagram has taken the world by storm because of its image-based services that allow users to discover anything from new travel spots to new products. Here, you can follow specific accounts to ensure you’re staying up to date with the newest products.
Look Through Online Marketplaces
Amazon, eBay, Walmart, GAME, Cdiscount, Alibaba and others – these online marketplaces are some of the best ways for you to find out what’s going on in the world of product buying. This is because they provide you with direct access to products, their prices, and customer reviews.
This is, then, one of the best ways for you to conduct research regarding how you should be building yourself and branding yourself. You also can use online marketplaces to find reliable partners for global expanding and sell products there.
Interact via Social Forums
Social forums can provide you with in-depth research and data regarding how users feel about different products and different niches. You will, then, be able to get information about the most trending products out there.
Not only this, but you can interact with users within these social forums. This will provide you with the opportunity to market and brand yourself in the process of finding information about the market.
Interacting in social forums will allow you to communicate with those who share similar values as you do. In this way, you can build connections that will help your business grow and expand in the process.
Characteristics of trending products to sell online:
- The product should be unique. Make sure that the product is unique to the market where you sell, or you can provide your customers with some additional features, new designs, or make some small changes to the product.
- Product isn’t widespread within the country. Make sure that the product is difficult to find or get in a short period of time so that your customers can get exceptional service in quick delivery, and get the desired product within their living area.
- The price should be affordable for the type of product you sell. Try to keep the pricing on the average range. Otherwise, people will think about the product value, and won’t make impulsive purchases. Also, they will look to other eCommerce stores.
- Sell a product that you personally passionate about. First, you will work harder, and try to deliver the product in the best possible ways, if you know its value, usefulness, and also use it personally. Most of the bloggers or enthusiasts started their business just from the idea. So, you can provide customers with some product that you know will be useful, tell about their advantages and see how demand is constantly growing.
Complete Magento Review 2019
If you are a business person, and you’ve been using various online platforms, then you must have heard of platforms such as Shopify, BigCommerce, and, Volusion, among others. However, do you know which is another popular platform among them? You guessed that right, Magento it is.
This platform boasts of over 250,000 merchants using it, and the numbers are continuing to grow. Well, this store building platform owes its popularity to the expansive feature set that it comes with, and also the ability to allow users to customize their stores according to their desires.
Roy Rubin and Yoav Kutner are the brains behind this fantastic platform. It was found in 2007 and sold in 2011 to eBay. In 2015, the platform changed hands again as eBay sold it to a group of investors. As we speak, Magento is currently being owned by Adobe since acquiring it back in 2018.
This review will highlight a few things you need to know Magento, including some of its best features.
Which of Magento eCommerce Packages Is Best Suited For Business?
Is Magento any good? Well, let’s find out. Generally, the Magento platform comes in two variations. Magento Commerce and Magento Open Source. They both serve for businesses. However, each of them will suit a particular business’ type and size. Let’s start by having a look at Magento Commerce.
Magento Commerce Overview
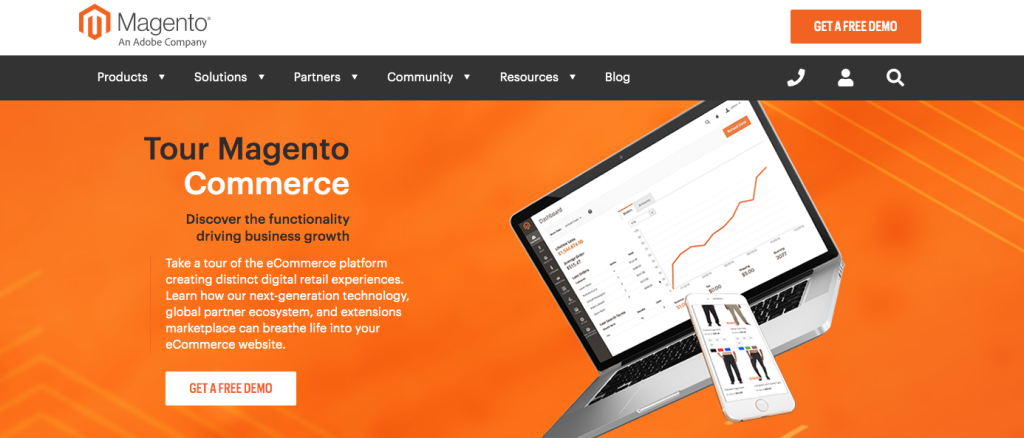
This is what was before known as Magento Enterprise Edition. It is a licensed version of Magento commerce services. However, it offers a lot more than just being a licensed version of Magento Open Source. This Magento pricing starts at $24,000 a year.
This edition was initially intended to be used by large-scale organizations that need a higher level of support and a broader set of functionalities. It comes completely packed with a set of great configurable features to improve the overall user experience.
A few additional benefits that come with Magento Commerce includes; offering technical support and dedicated account management. It has a few unique features as well, such as Magento Order Management, Magento Shipping, and ElasticSearch, among others.
This is why you will have to incur the Magento enterprise cost. The edition is also divided into two different versions: Magento Commerce Pro and Magento Commerce Starter. Magento Commerce Pro is a right solution for retailers looking for an Enterprise Cloud hosting services combined with the Magento Commerce license. The pricing of this version starts at $3,399 per month.
The Starter edition, on the other hand, comes with a pricing of $1,999 per month. It can be used by those looking to combine their Magento Commerce license with a Cloud Hosted infrastructure at an affordable price.
There are also a few differences between the two. For instance, the Pro edition comes with business intelligence features that can give you full access to your data while the Starter edition doesn’t have these features. They can, however, be added but at some additional cost. Let’s move on to another Magento 2 package review.
Magento Open Source Overview
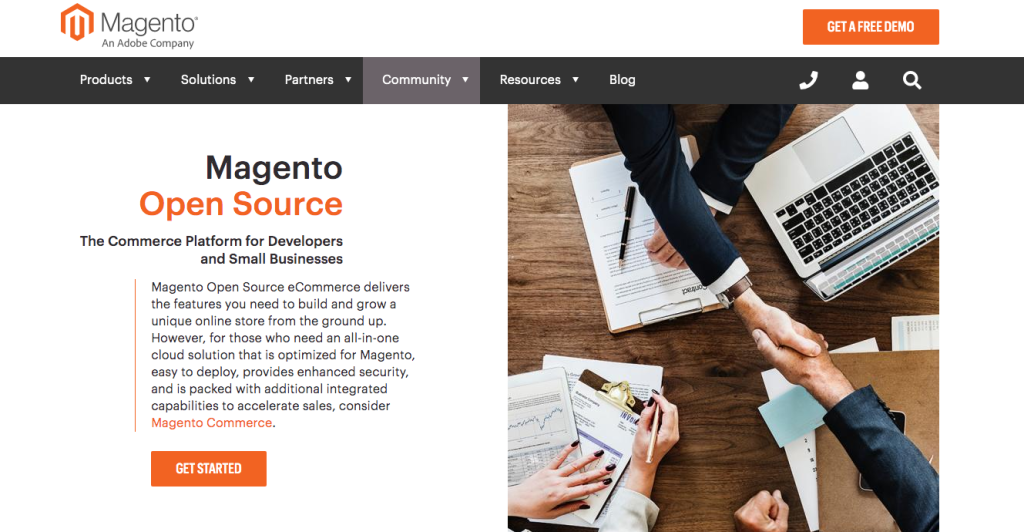
This Magento costs nothing. It is entirely free to download and get built-in basic functionality. This Magento open source edition was introduced back in 2008 and was known as Magento Community. As the name suggests, this Magento version is free of charge and therefore available for everyone to use.
It also allows users to install and use some extensions and make some adjustments during the configuration of the software. This offers a chance to the users to customize it and use it according to their desires. The Open Source edition makes a good solution to startups or growing businesses due to its ease of use.
It allows users to build eCommerce stores quickly and comes with a variety of great tools to improve user experience. However, Magento has always been working with a very talented team of developers to bring new extensions, adding to what it already has.
This makes it even much easier to use the software as it adds more functionality to it. More about this can also be found in an exclusive Magento community review.
Features of Magento eCommerce Platform
To understand more about the software, let us see some built-in features it comes with and why they make great Magento solutions.
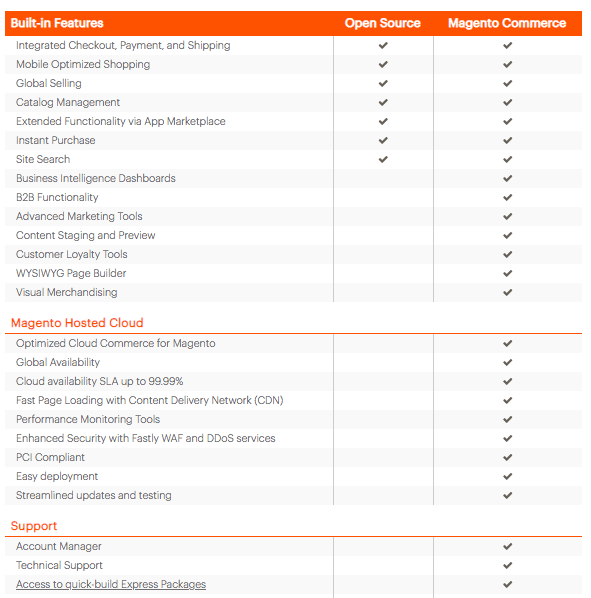
1. Inventory Management
Among the things that make Magento so easy to use is its ability to let you control your online shop. Through the dashboard, you can access your inventory as well as your orders.
Furthermore, you can add products and put them into different groups, categories, or bundles. It also allows you to keep track of your inventory, create nice templates for your product, and various business models such as digital goods, services, and subscriptions.
2. Coupon Management
Magento platform makes it easy for its users to entice their customers by allowing them to offer great deals to the customers. Some of these great deals usually involve purchasing items at a discounted price.
Aside from discounts, users can also use the coupon management feature to create coupon codes that people can use in your shop. This will allow you to apply discounts on a few selected items in the store. This helps in capturing your visitor’s attention and most influence their decision to buy something.
Log in to the backend of your shop and configure your default coupon settings. You can also access the Cart Price Rules if you want to edit your existing coupon codes or when creating a new one.
3. Multiple Payment Gateway Options
To make your business even more efficient, you can integrate multiple payment methods with your online shop. This can be done in your admin settings simply by putting them on or off.
This is a great feature as it also allows different customers to choose their desired methods of payment. The various payment methods include Authorize.net, PayPal, WorldPay, and CyberSource, among others.
Useful for you:
4. API for Web Services
Magento has a web API feature that allows the users, through developers, to create and use web services that can interact with the Magento software.
These web APIs are often used to perform various functions including; ERP, CRM, and CMS integration, creating a shopping app, and also creating Javascript widgets to use in your storefront or your admin dashboard.
5. Customer Groups
This is also another great feature offered to help its users to manage their targeted customers. This feature helps when it comes to marketing your products.
It allows the users to control their visitor’s user experience for different customers. You can also categorize your site’s customer base into different groups.
You can then start creating rules, taxing classes, and grouping specific discounts depending on the individual customer group.
6. Product Bundles
When you make it fast and easy for your shoppers to find the items they need, your sales can greatly improve. This is why Magento offers its users the ability to create groups of items that are related.
This is achieved through product bundling. It can simplify your customers’ choice, thereby positively influencing the shopper’s decision to buy a product.
This feature enables you to create custom bundles and let the visitors create individual bundles of their own from the options you had already bundled up. Apart from boosting your sales, it also improves the customer experience.
7. Built-in SEO Features
When doing business online, you know the importance of staying at the top of the search engine. This plays a very key role in terms of sales conversion. This has been a challenge to most online stores but not when you are using Magento. The platform comes with built-in SEO features to help you get started with the basic requirements of on-page SEO. You can configure your SEO settings also at the admin panel.
8. Marketing Tools
Marketing is always a key factor to consider for a business organization to become successful. Depending on the marketing strategies you use, proper marketing can bring a huge difference when it comes to sales.
Magento has a few selections for their users to strengthen their marketing sector. These tools focus much on two areas. The first one is to help you expand your brand awareness and reach. This enables users to make more sales conversions by creating more opportunities.
The second focus is on helping you to get your customers to spend more. This will increase your average transaction value. With all the marketing tools provided, you can increase your profits and steer your business right to success.
9. Site Management Features
The site management features are those that Magento has enabled you to control multiple stores and sites from your admin panel. They also allow you to customize templates to achieve your desired designs.
They also support multiple currencies and languages for smooth transactions. When using the Magento platform, you don’t have to worry about robots trying to access your site. You can keep your site safe using the CAPTCHA functionality.
These features also make it easy for users to import and export catalog and other customer information.
10. Newsletter Management
This allows you to create and manage mailing lists within the platform. With these features, you can allow your visitors to subscribe to your newsletter from the account, product or checkout pages.
The newsletter management features make it easy for you to categorize your customers and manage their information based on their order statuses.
The features also help your marketing efforts as they allow you to run targeted advertisements or promotions according to your subscriber groups. In general, these features are meant to improve your business through email marketing.
11. Order Processing
If you want to run a successful business, then you must prepare yourself to handle a lot of sales orders. However, since most order management systems are often not that much powerful, Magento lets you use its amazing order processing features. This makes your order fulfillment easy and fast.
The process involves three main stages. You will first need to log into the backend of your shop and see if you have any pending orders.
If you do, the second phase involves carefully examining the following sections; address information, order and account information, ordered items, and the preferred payment and shipping method.
When all of this is done, you can proceed to complete the order total section, and that’s it.
Useful for you:
Magento Themes and Designs
Magento gives you a platform to build your store and allows you to use its templates. When you use free themes, you will notice that they are quite basic or have minimal functionalities in their designs. However, to set up plain Magento 2 store, you can use the Luma theme which is offered by Magento. It is great for a start, and gets you covered with basic functionality for the store.
But this shouldn’t be why you can’t have a beautiful store with amazing functionalities. You can always reach out to the paid versions of the Magento themes and designs as they are quite flexible and come with many functionalities. You can check Magento Marketplace or any other website like Themeforest that offers themes and templates, and choose the one you like by style and features.
These themes can also be customized according to the user’s desires to improve the visual options and the general layout of the design.
Magento Services and Support
Magento Security Services
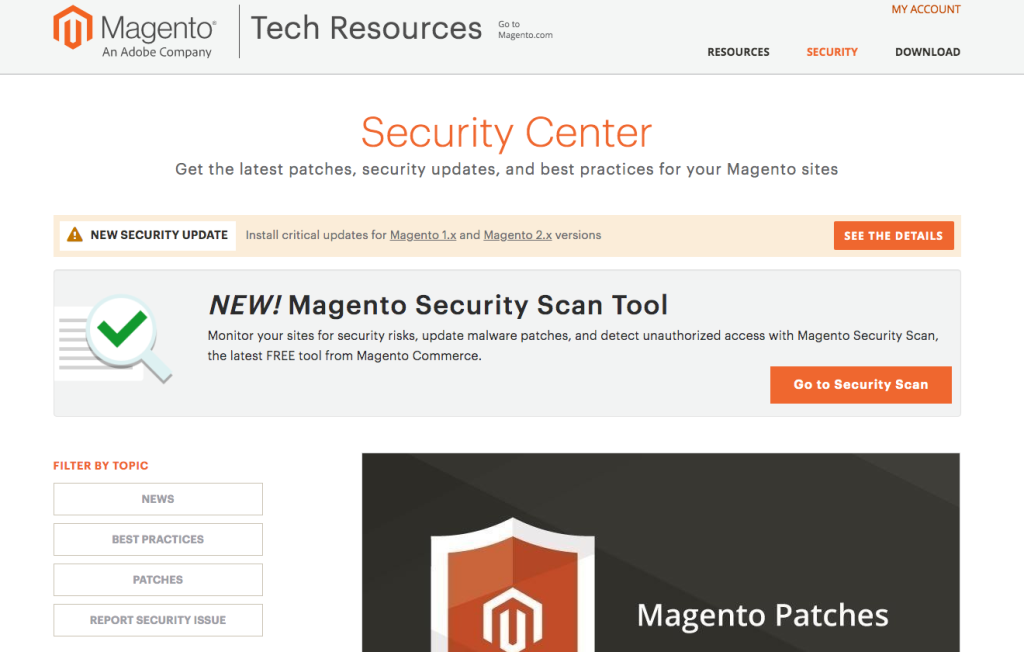
Besides excellent documentation guidelines, Magento offers a free security scan tool to detect any glitches and update patches at your store. Also, they provide security services that cost form $1,000 to $5,000 and solve SQL injection vulnerabilities. When you just launched your store, Magento gives you PCI compliance and two-step authentication plus Google reCAPTCHA to protect your business and visitors from any system and data attacks.
Magento Knowledge Base
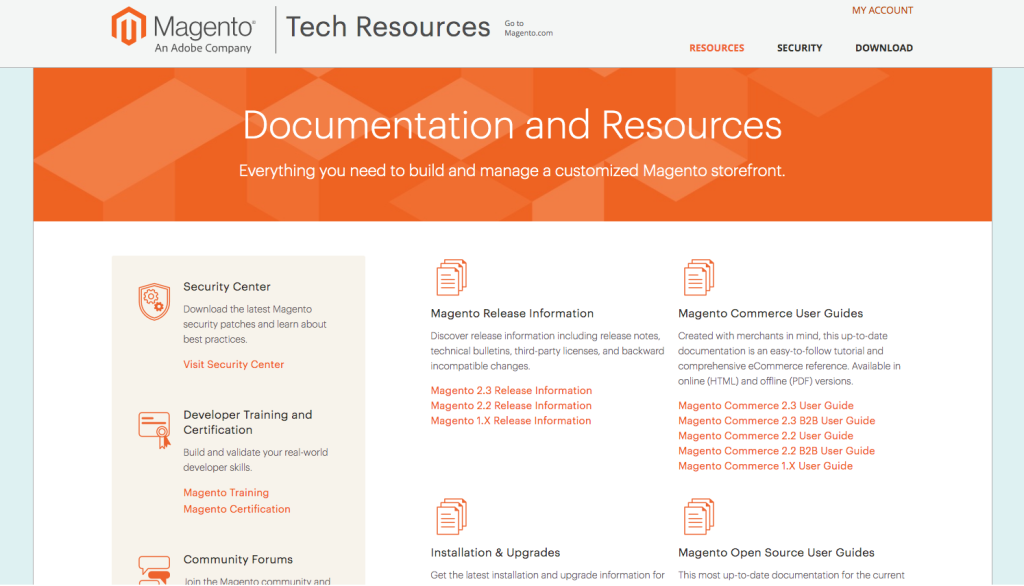
For their customers, Magento has prepared an extensive documentation base along with useful guides on their products, and latest eCommerce trends. If you want to check the current version tech stack and features, or to learn how to increase the efficiency of your store, you can refer to the tech or library resources.
Among other useful resources that you can find on the Magento site are the following:
- User Guide
- Magento Community Forums
- Blog on eCommerce and Magento Usage topics
- User Guide for Magento Marketplace
- Resource Library: Webinars, tutorial videos, topical guides.
- Support: For sales and general info, but not specific tech support questions.
- Training courses & certification at Magento U.
Pros and Cons of Magento eCommerce Platform
Pros of Magento
Below are some few advantages you can enjoy when using the Magento eCommerce software.
User-Friendly. As mentioned before, this is an amazing feature-rich eCommerce software and a quite popular one for that matter. These features are great for better performance.
Scalable. Well, for those merchants looking fort a scalable eCommerce software, try Magento. Clearly, the reason why most top businesses use it and currently hosts over 125,000 sites.
Feature-Intensive. Magento has a lot of amazing features that make work much easier when it comes to store management, among other things.
Cons of Magento
The software also has a few shortcomings. Below are some of them.
Costly. It becomes a bit expensive to access various features with tons of functionalities. For your shop to keep on working efficiently, you need to invest. This means parting with some extra bucks. Maintaining such a great platform also comes at a cost.
Time-Intensive. Generally, Magento is an amazing business software to use when getting into the eCommerce business. However, it can be quite challenging and time-consuming, especially when moving from one version to another.
Magento is a force to be reckoned with when it comes to eCommerce platforms. It comes with great features and tools to help you build a store, add products, manage it, and make profits. This is an awesome software to use if you want to own an online store.
How Important Are Gift Cards for an eCommerce Store?
An eCommerce store aims to rack as much as products on their virtual shelves to suffice the needs of every single user visiting the store. Despite hundreds of products, a buyer may still be unable to buy an item suitable to gift a friend or family member on a specific occasion. All your efforts of managing products in multiple categories drain out when a user leaves your website because there was nothing worth mentioning for gifting the best buddy.
The idea of creating gift cards pops in as an alternative. It encourages the users to buy a shopping voucher to gift it and let the receiver shop his or her favorite items. It is important for any online store that does not want to lose the customers. It has many other benefits as well that I will discuss later in this post.
What Is a Gift Card?
A gift card is a win-win option. It is a prepaid card, which a user buys to send as a gift to someone special. The eCommerce store makes a sale, and the buyer gets a brilliant gift to send. The receiver gets a voucher to shop for his or her favorite items free of cost. Therefore, it is a blissful choice for all.
Useful for you:
How Does It Work?
An eCommerce store may not have the functionality of selling gift cards in addition to their catalog. They can seek assistance from applications such as Magento 2 Gift Card extension or any other tool suitable for the eCommerce platform they use. The extension helps to create multiple types of gift cards and displaying them on a dedicated page. It has three different pricing models to set the price of the gift certificates and many customization options.
How Important Are Gift Cards?
Gift cards have multiple implications on the sales, branding, and promotions of an eCommerce website. The intriguing fact behind this strategy is adding a gift option that prevents the users from leaving the store with an empty cart. The post discusses some more reasons below that make gift card important and value addition to your online store.
1. Retaining Your Existing Customers
A gift card is an additional option for your existing buyers to shop around. The customers can simply buy a gift voucher if they are confused about buying a gift suitable for their family or friend’s interest, or need to attend a gathering or a special event.
We all want to present something precious to our friends that they need the most. However, somehow, we may not wholly know their needs and interests. Therefore, to relief your customers in selecting a gift, let them buy a coupon card so that the receiver can redeem it on your store and order his or her favorite items.
2. Outreaching New Buyers
Every business aims to expand beyond a locality and customer base. To attract more customers towards your customers, the creation of gift cards is an effective strategy. Your customers who purchase the gift cards would like to send it via email, personal message, or by post.
The gift card receivers are your new customers as they are recommended to make the purchases from the store. They visit your store in pursuit of turning in the vouchers, and they find valuable products or services at your store. Once they like your store, they will come again. You get the new buyers while retaining them depends on you.
3. Building Trust and Credibility
The way your customers send out gift cards to their loved ones, it serves as a recommendation. It is a form of testimonial that I trust the website, and you must try it with a few purchases with the coupon.
The receiver of the gift voucher may surely discuss and appreciate the items he or she receives in return on the voucher. This helps you build trust and credibility among the potential customers and their social circles.
4. Collecting Funds Before Taking Orders
Gift cards are prepaid. The customers pay you in advance and refer your store to the gift receiver. Therefore, you get the funds or the revenue quite before making a sale. Having funds in advance improves the cash inflow of your online venture so you can make more items available in your store.
5. Adding Another Stream of Income
You might be making sales and earning money from the traditional buyer’s journey. In addition to routine shopping, online buyers are always in search of discounts, coupons, and vouchers. If you are not offering gift cards, you might be losing part of the income that you can receive from them.
Your customers may visit the store once or twice a month to find if you have an appealing deal to offer. With gift cards, you can encourage them to make the purchases and save money. You can create discount vouchers that allow the customers to shop more while spending less of their money.
6. Free Branding and Promotion
The gift cards bear your brand logo, business name, and any slogans that you have. With further personalization, you can add images of various products or business premises to the gift cards. You can endorse a social cause or condemn the social evils on cards, and people will connect emotionally to your brand.
They will get to know about the good things you care the most. As a result, they will start recognizing your business as a brand. The more a gift card spreads across the buyers, the more they promote your venture.
Filippa K Complete eCommerce Store Review
Learn how to elevate the success of the store with minimalistic design and powerful brand’s philosophy.
Filippa K is a Swedish clothing brand. It was founded in 1993 by Filippa Knutsson and has grown to be one of Sweden’s leading fashion brands. The company is based in Stockholm and also operates a growing number of profile stores in Scandinavia, the Netherlands, Belgium, Germany, and Switzerland.
Minimalistic design, sustainable philosophy are what make Filippa K strong and unique clothing brand. Their dedication to high-quality fashion-wear is impressive. However, what makes Filippa K even more different is their responsible attitude to sustainable fashion and manufacturing. Taking leading positions and awards in sustainability, they empower their customers to build a durable wardrobe with qualitative, luxury, and timeless garments.
Looking at the successful example of Filippa K brand strategy, we want to make a review of their store, and show you the essential features for the clothing store website to consider. Let’s move on to the store functionality.
Website Overview
The first impression when you enter the site is pleasant. You see the minimalistic image, the main banner with the recent collection, and navigation menu with all the categories.
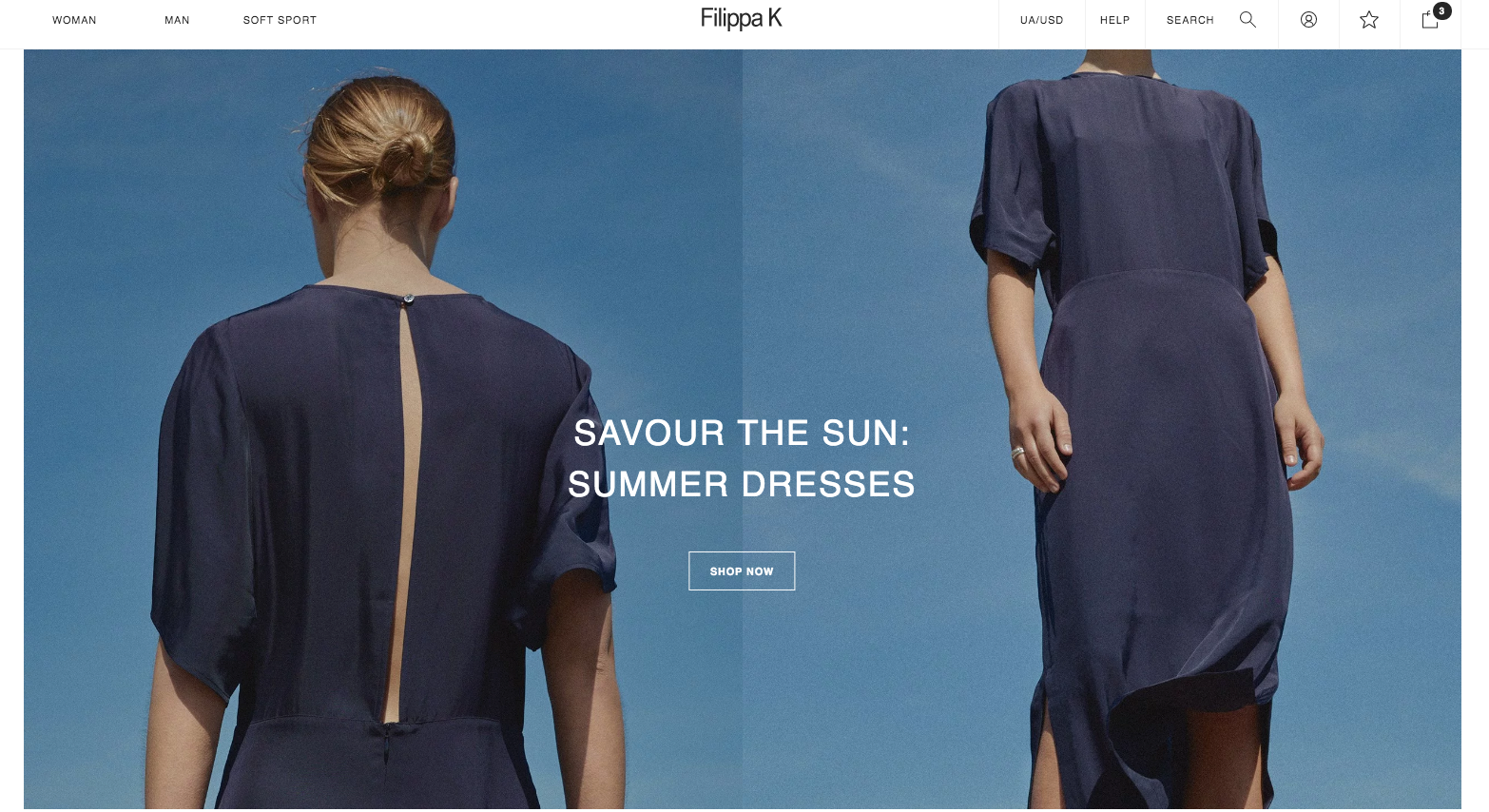
After you check all of the categories, you notice that all the pages are performed as a sidebar which is convenient for the process of making buying decisions.
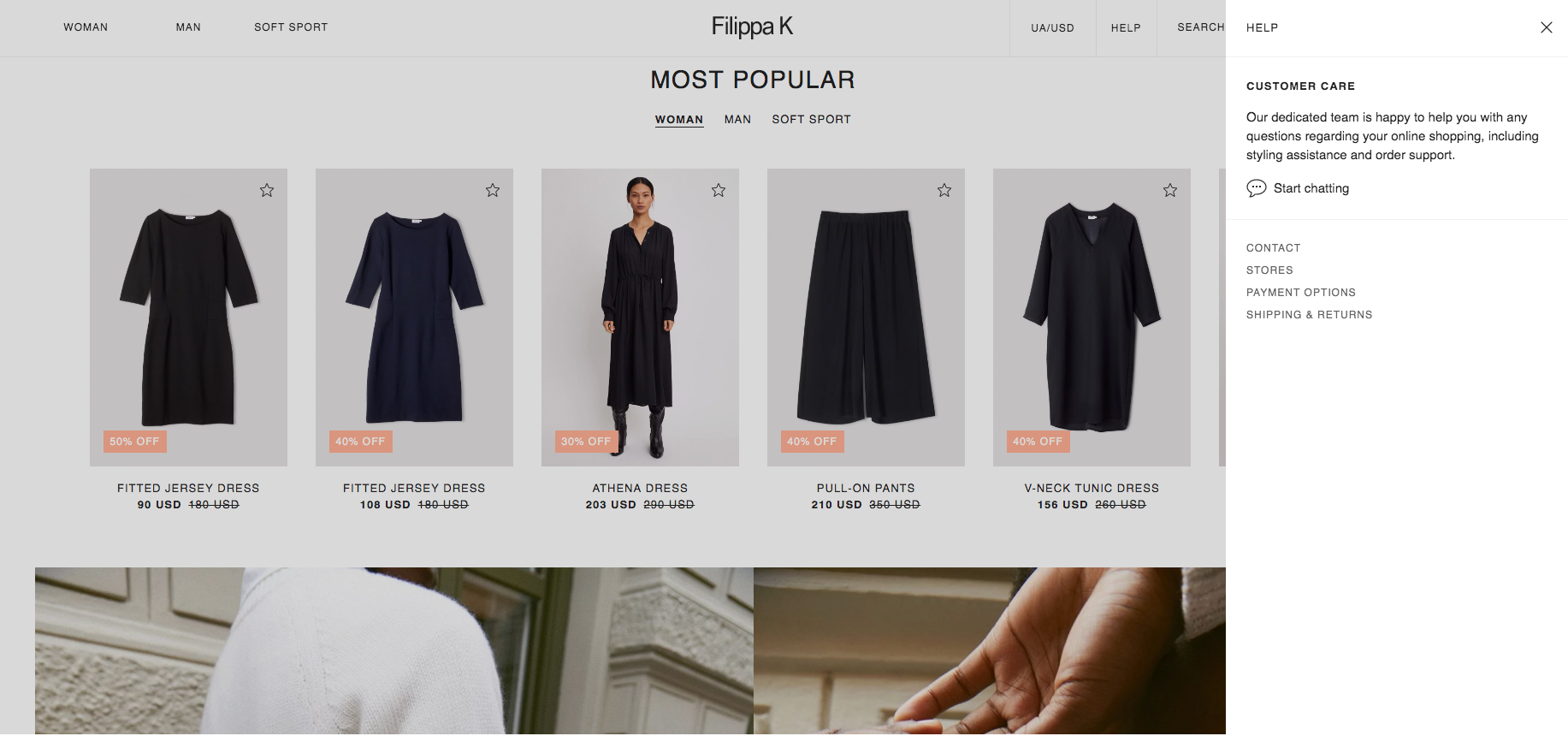
A customer can reach the information in several clicks, which proves excellent usability of the site.
Now let’s move on to the store functionality. We will explain the important things to consider for your store if you want to provide the best customer experience and keep your business on track.
Functionality
Filippa K store consists of:
Filippa K has a simple, yet convenient site structure. A customer gets clear navigation within the store, which we will review below.
Desktop
Home Page & Navigation
Navigation plays a key role in building great UX. It requires to place the most important information and featured products on the main pages. The navigation menu of Filippa K is simple, minimalistic, which is great and doesn’t distract the user. This lets customers browse and shop easily because clean design with minimum sections cuts off the time for a search of the particular item.
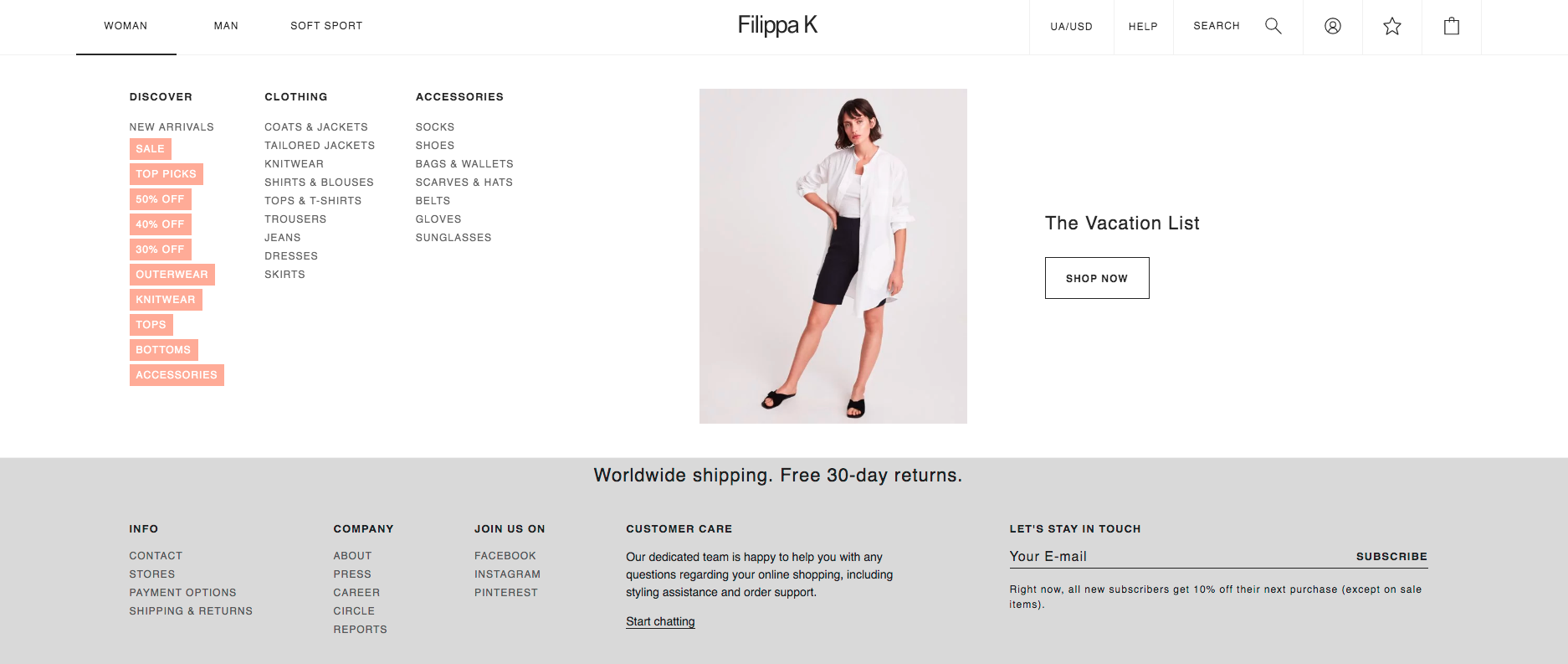
In general, Scandinavian design is quite popular worldwide, and it can be adopted in foreign markets.
A header has three product categories:
- Men
- Woman
- Soft Sport
The minimization of the number of categories makes it easier for a customer to find the target product.
At the top of the page above the header, you can see the line that says free shipping, or some promotional information. This spot is called Top Promo Banner. It will help you to show more of your new discounts, and let your customers buy more products and try out hot deals with some promo codes available.
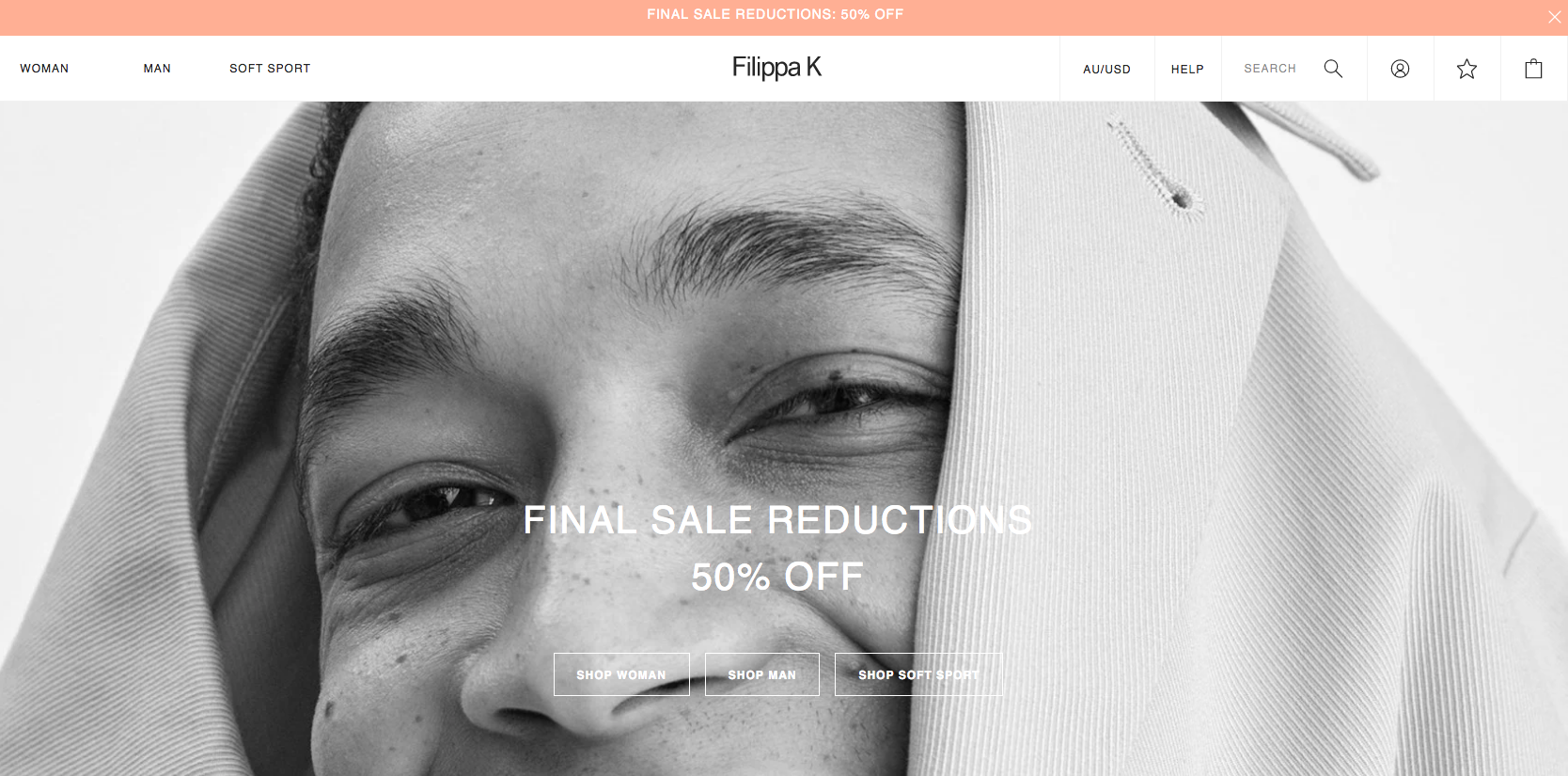
This will increase customers’ loyalty and attract more visitors to your store. Filippa K has Top Promo Banner that tells about free shipping and returns. You can add this block to all important pages and get the most of it.
Below the header, you see the banner with product categories. The banner has main categories of clothing to attract their clients to these pages. We also notice an impressive feature called shown in the image. You simply click on the number at the right corner of the image and get a sidebar with the information related to the products shown on the picture.
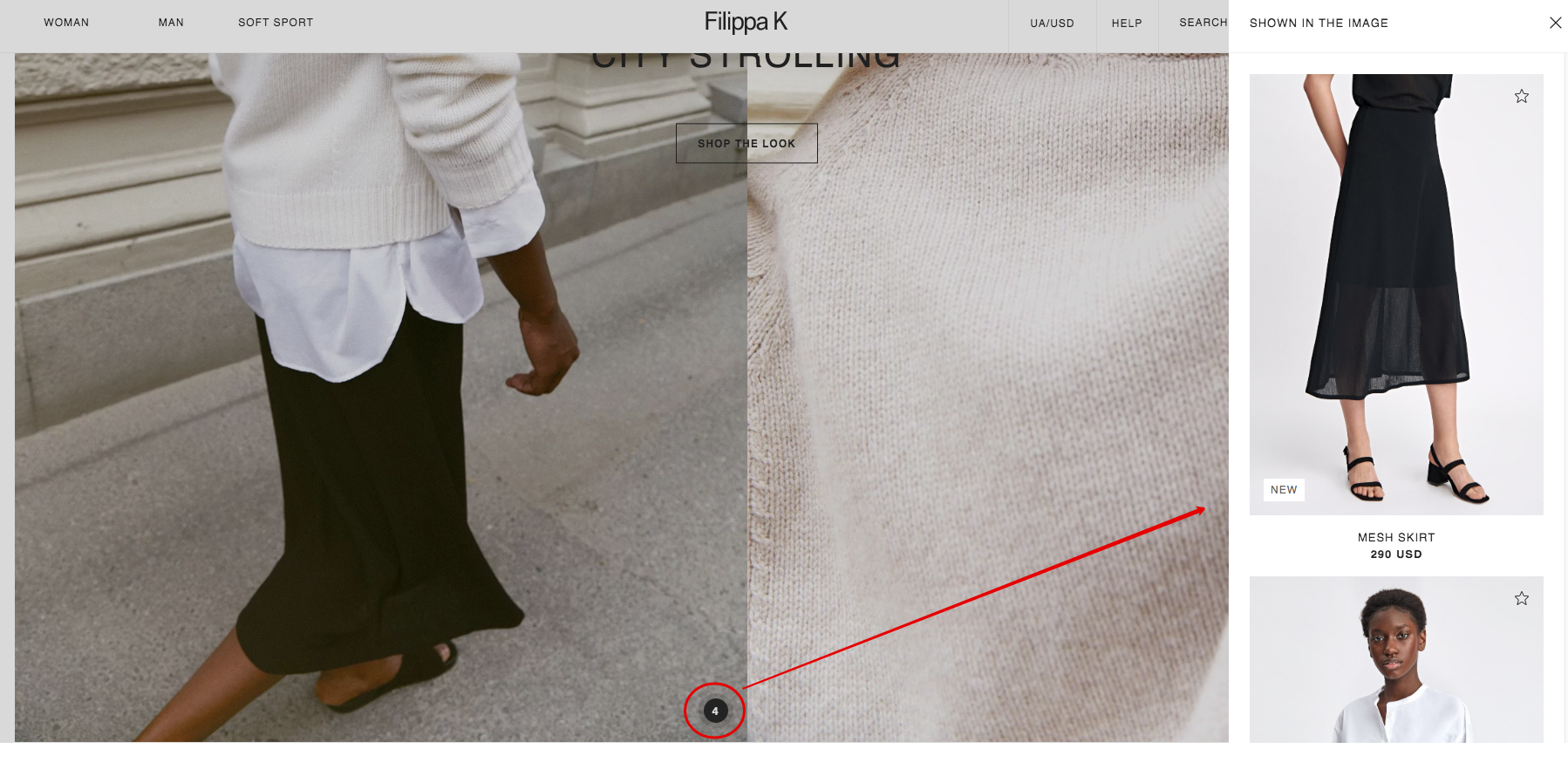
Also, this lets Filippa K showcase featured collections and their vision for all customers that come to their site and gain a foothold in customers’ mind.
Product and Category Pages
What is one of the most crucial in any store besides convenient navigation? Of course, beautiful, detailed, and qualitative product presentation. All of it you can find at the category and product pages.
When you enter one of the categories, you’ll appear at the category page with all products’ range. Filters are performed in a standard way when you can select some parameters from the list, and apply them to find the exact product. However, filtering could be improved by making the selection of parameters instant without page reload. This will save time for search of a product.
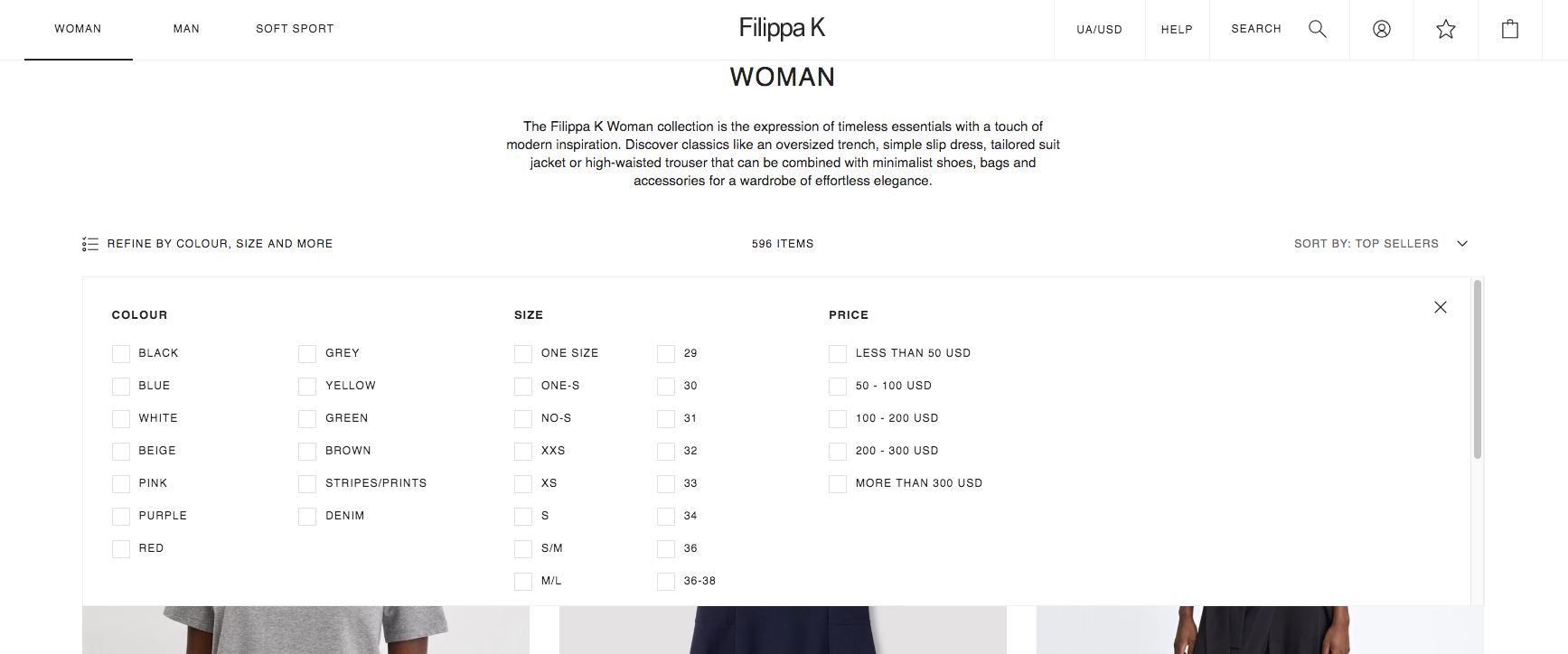
Filippa K has a high quality of product images that let the user see the product in a better way. Another benefit of the product listing is that you can make a quick order by choosing the size, and then your product automatically appears in cart. Also, the ability to select a different color without going to the product page will make interaction with product smooth, and increase the chances for a purchase.
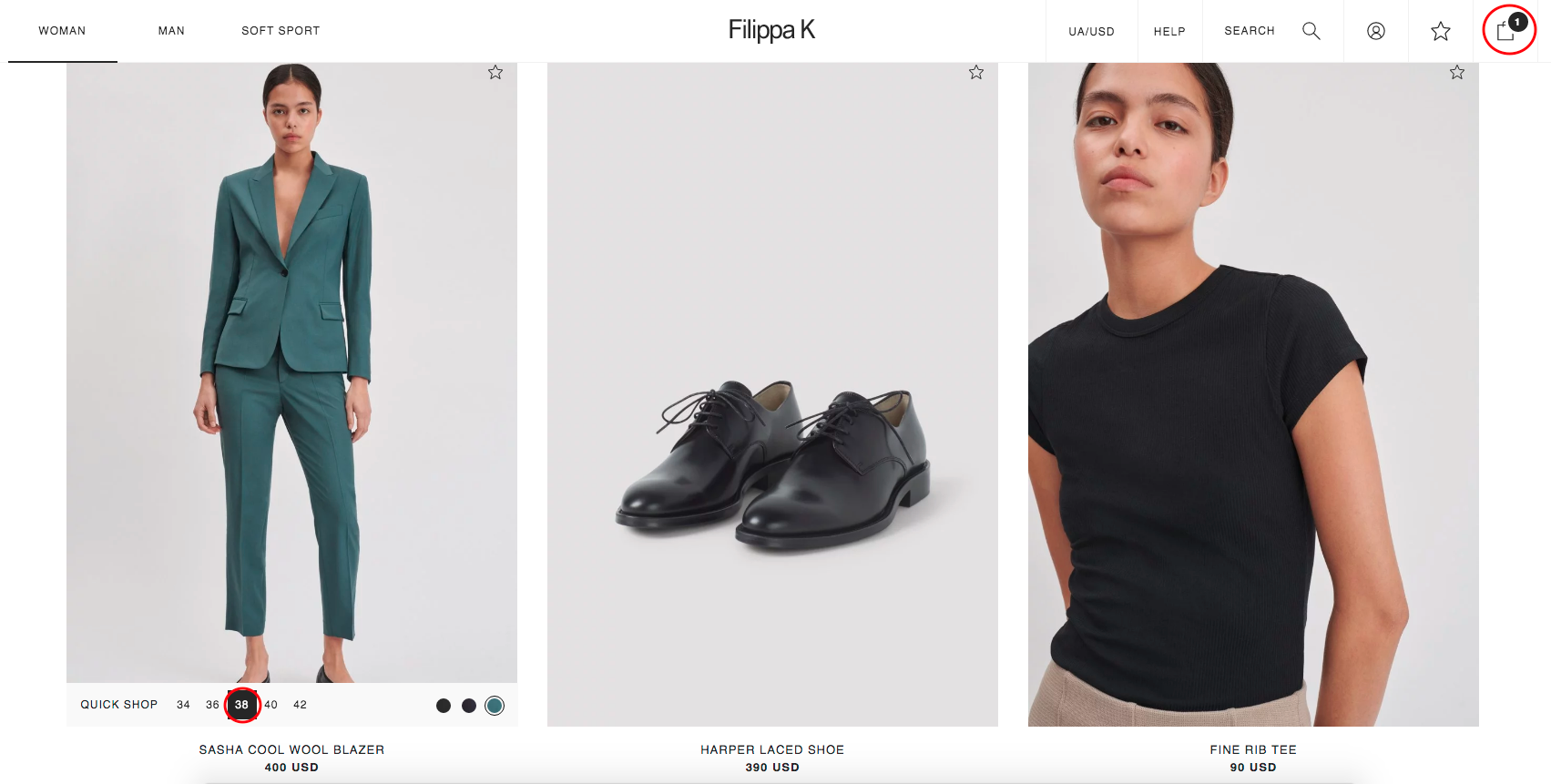
Each product has its page with a description, product images, Swatches, Select Size and Size Guide, plus Add to Cart and Add to Wish List.
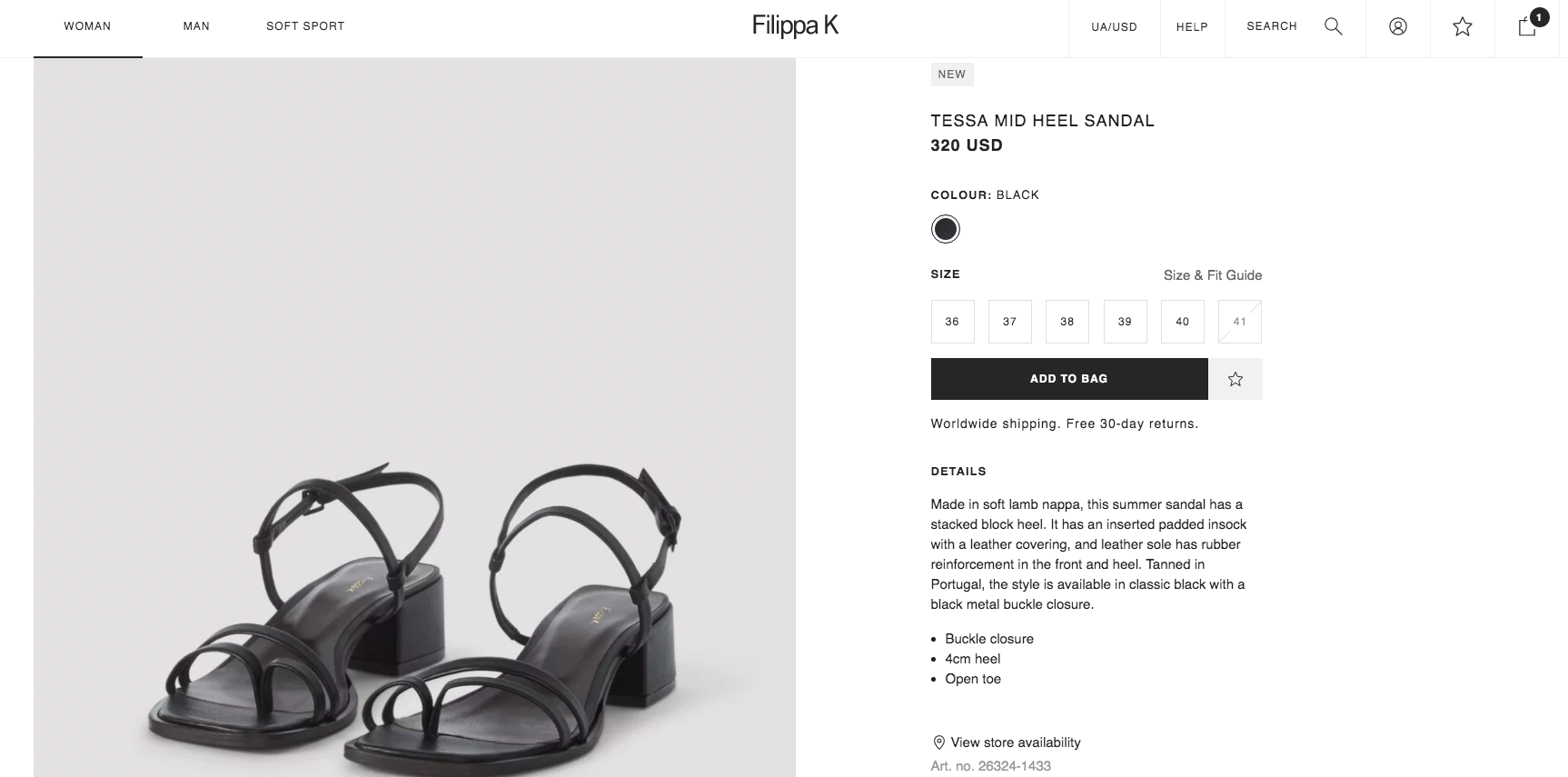
Also, a product page has detailed information not only about material & care instructions and general description, but also product origin information. This proves that Filippa K has transparency in their manufacturing processes, and gives a customer even more information about all stages of the production cycle. It creates trust and close communication of the brand with a customer.
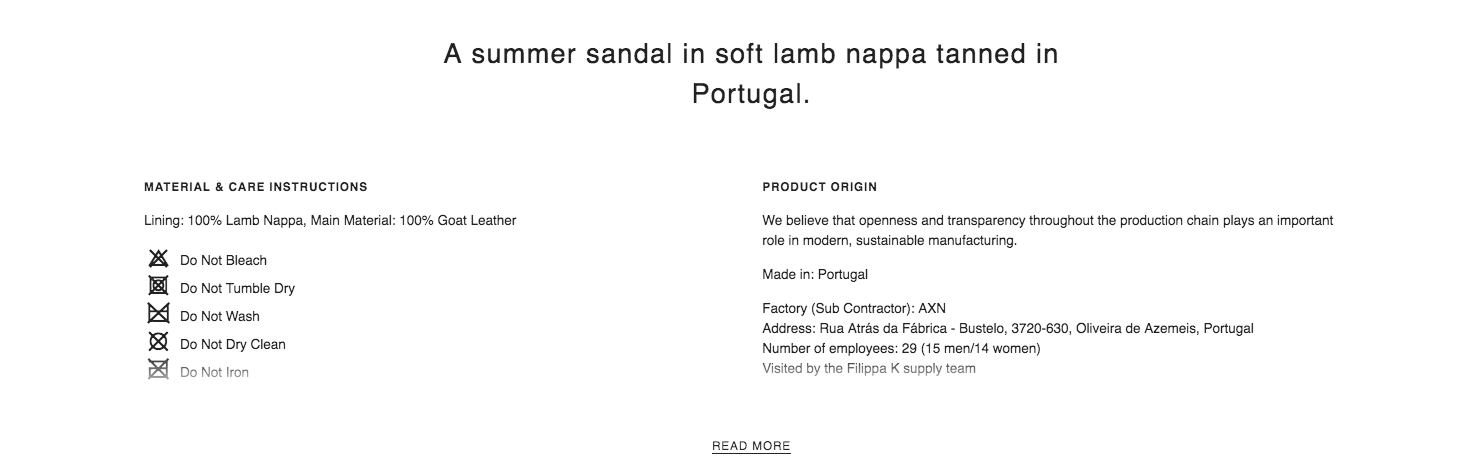
Filippa K has an additional feature called Wear With. This attribute is needed to link the product with other matching products from the store. That’s how you can assure cross-selling. Also, if you scroll to the bottom of the page, you still can order the product by selecting a size/color, and clicking the Add to cart from the pop-up window. This is very convenient in case if a customer sees other products but still remember about the initial product on the page.
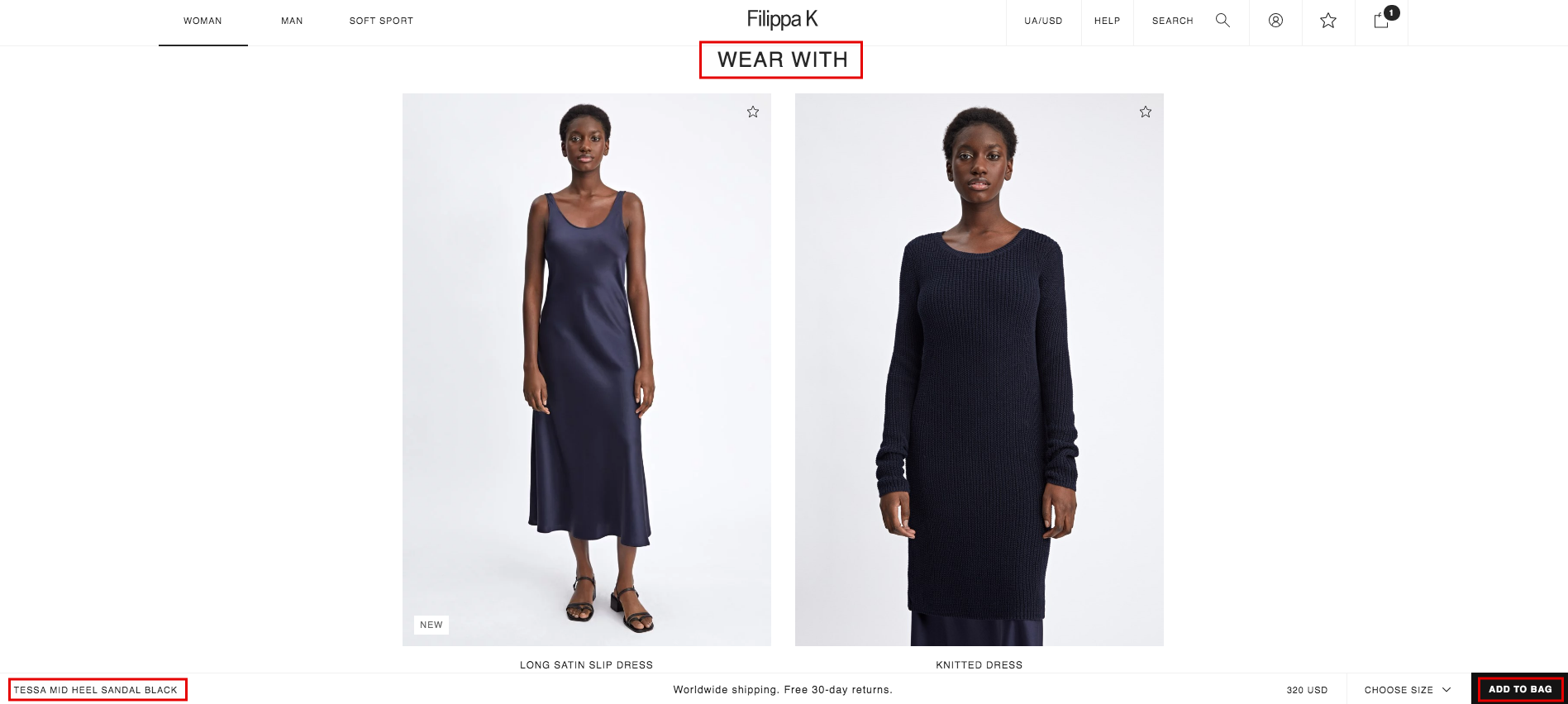
After a user added product to cart, the page goes back to the top, and a customer sees You may also like block. It helps to show similar products to the initial one or to present popular items at the store. In the case of the selected product being out-of-stock, a customer can choose a similar one from the feed and still make the purchase. Then a customer needs to click on the shopping bag to go to the Checkout page to complete the order.
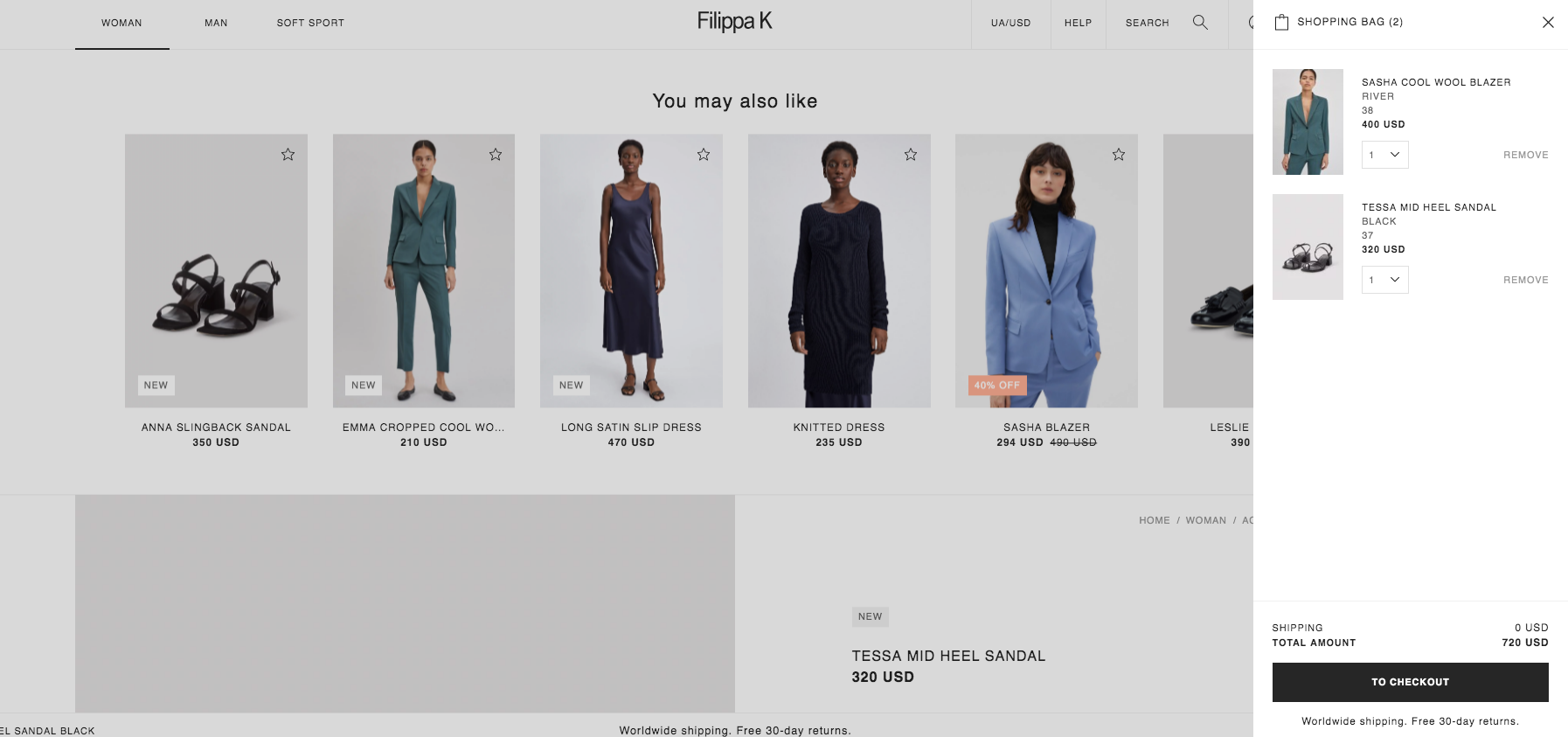
Checkout Page
Customers often want to buy the product quickly without filling out lots of information. That is why most of the stores and Filippa K isn’t an exception, prefer to use One Step Checkout. It requires to fill out the information on one page and then redirect you to the order summary.
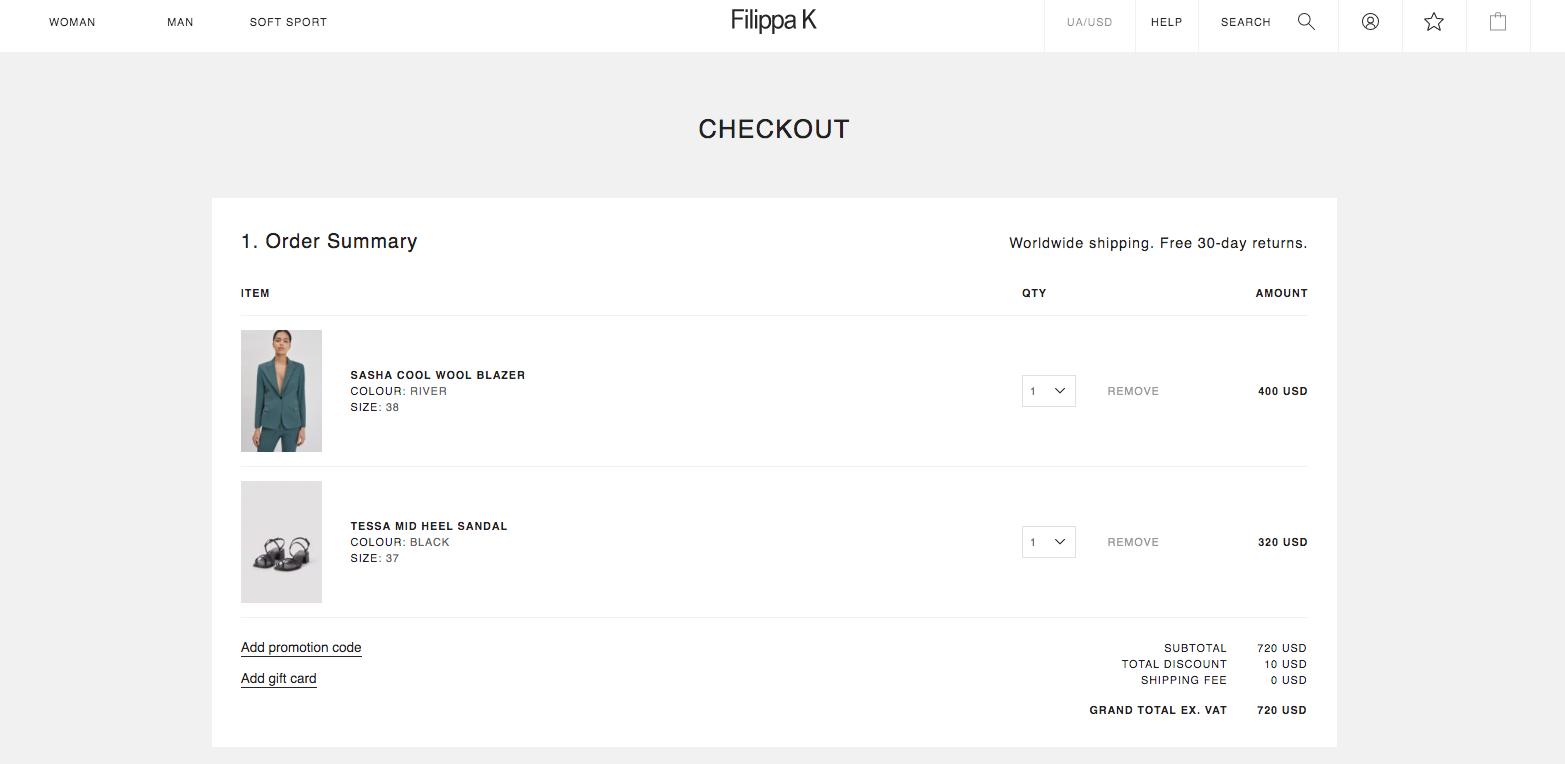
Payment Methods
Filippa K store has two payment options:
- PayPal
- Debit/Credit Card
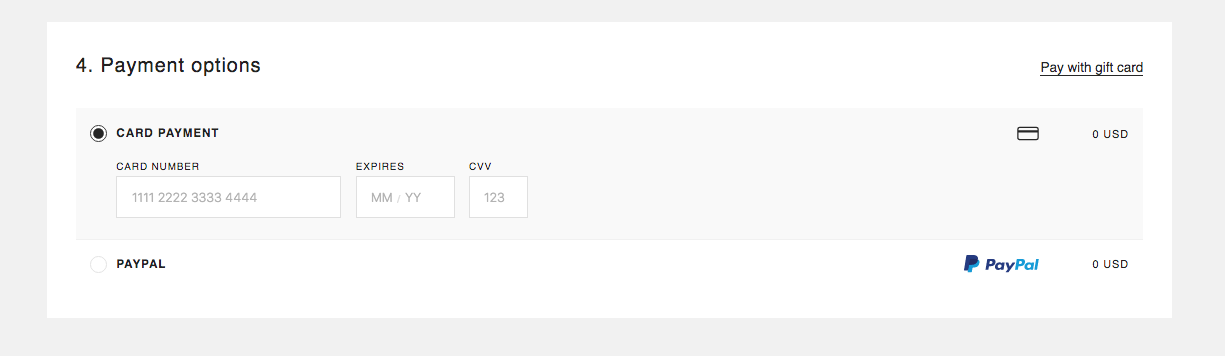
These payment types are suitable for most of the clients. However, integration of Split Payments, Store Credit, Checks will give more options for the user to complete the order. So, if you are looking to make the best user experience and increase your sales, then consider making a wide range of payment options.
Marketing Kit
Here is the list of useful marketing pages and features for customers’ convenience:
- Store Locator – helps to find the nearest store.
- Filippa K Circle – a company’s blog that has articles, annual reports, activities, charity and sustainability campaigns, and plans. This lets Filippa K to build their name and to get acquainted with their audience with the brand and gain people’s loyalty. There are several topics about sustainable manufacturing and consumption, and also interviews with like-minded people.
- Sustainability at Filippa K – this page includes the explanation of how Filippa K works to produce recycled garments, their mission, and a result that you can check in their latest report.
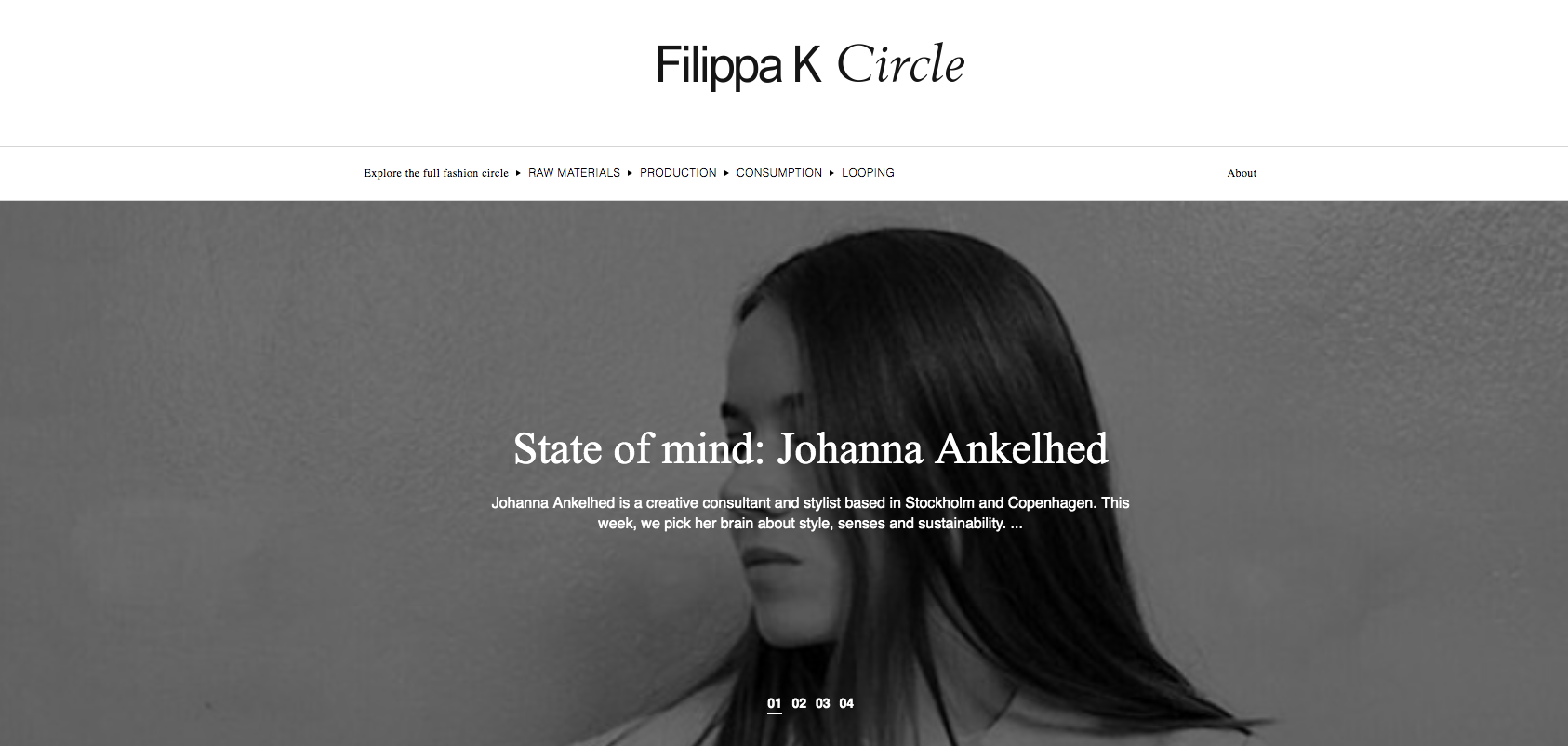
Those features help clients’ find relevant information about the company, location of stores, manufacturing process, and learn more about the brand.
Now, let’s explore the mobile website look and feel because the tendency of shopping from mobile devices is continually growing. More customers are choosing to buy with their phones because they can do it from any place and on the go. Improved mobile shopping experience also increase the number of orders at the store.
Mobile
If we take a look at the mobile version, we see that the navigation style is simple and handy. You see all the categories as well as promo information.
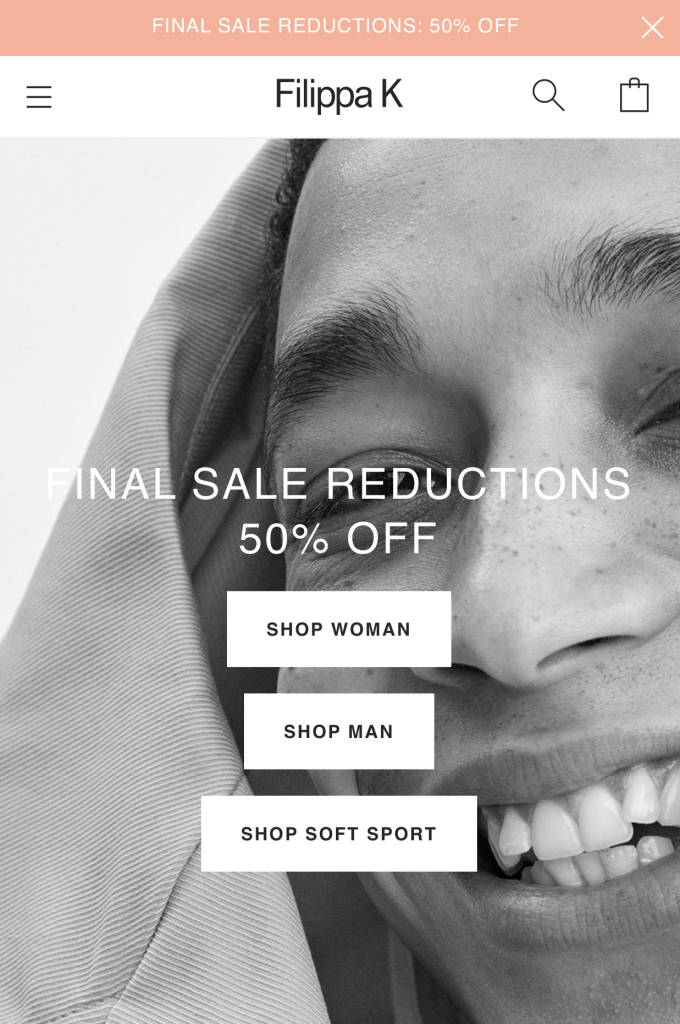
The menu is also informative and repeats all the categories that the desktop version has.
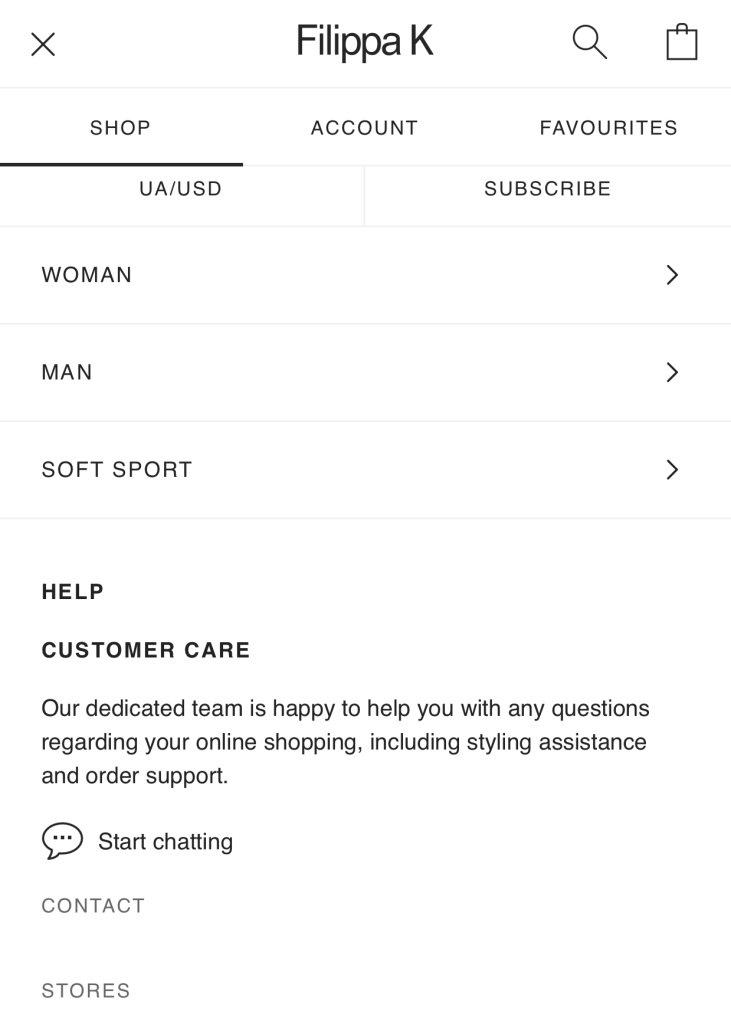
If we click on the category, we are lead to the product listing. Filters are at the bottom of the page, which is unusual. They made 2 columns to select from clothing parameters and sort by price, brands, and date of publication.
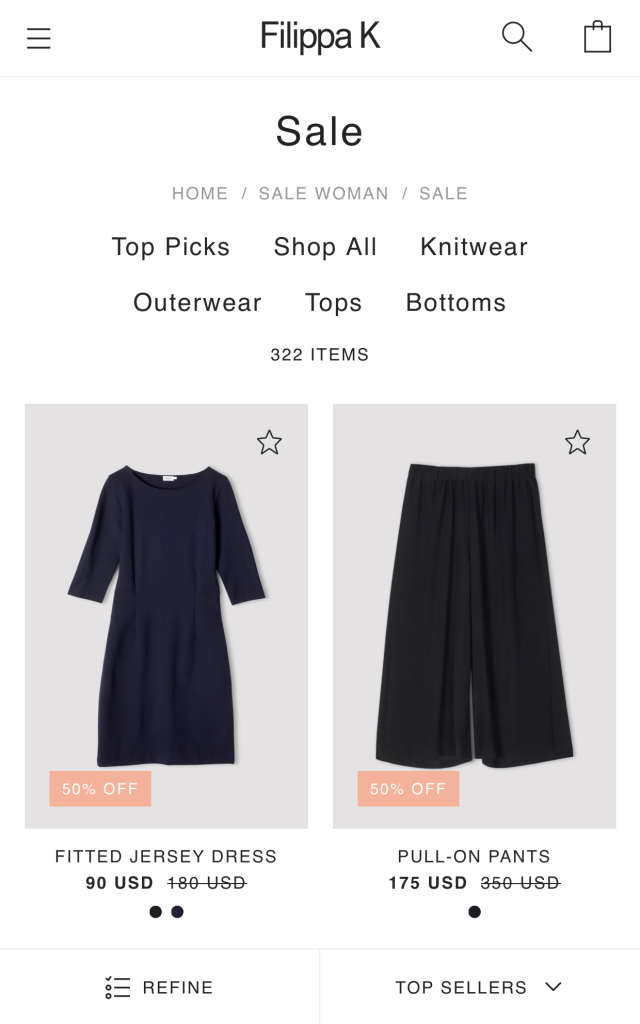
However, the mobile version doesn’t have a quick order feature due to the difficulty of clicking to the small description area.
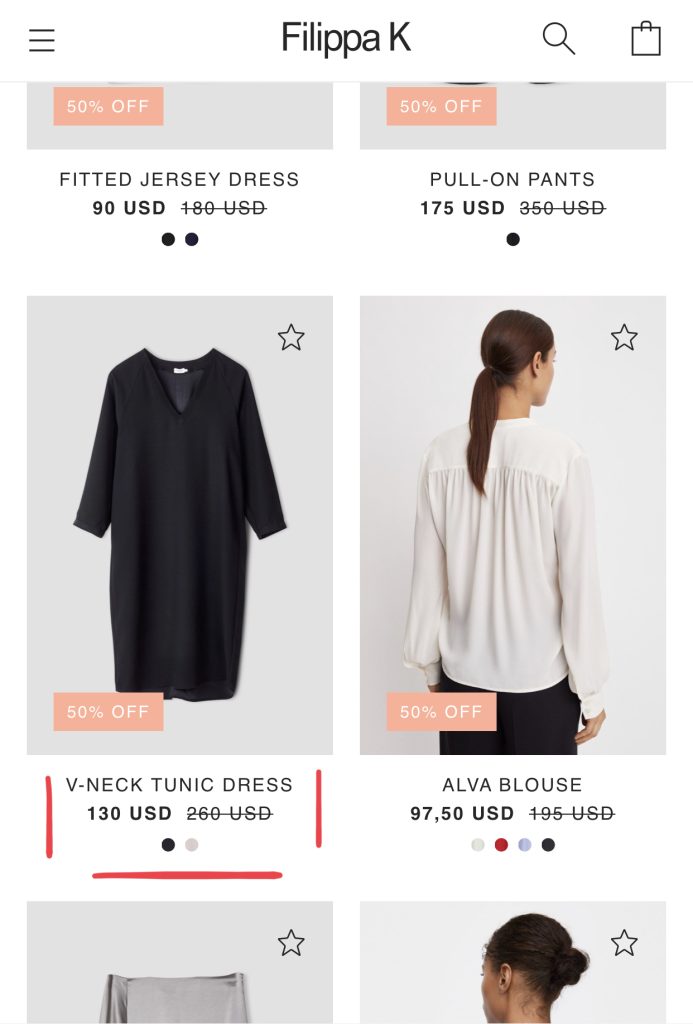
Below in footer, you have all the site categories that you can choose easily from the list.
The product page provides all the details such as images, description, reviews, related products. It covers everything the customer wants to know before they choose to buy a product at the store.
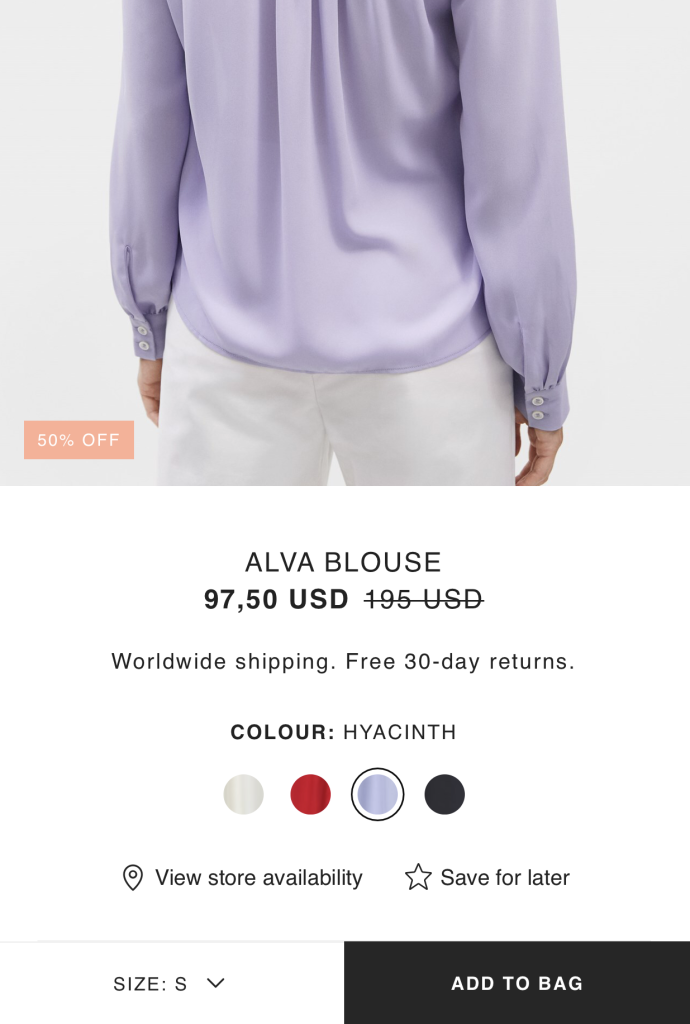
This page also has one more useful feature, which is called View store availability.
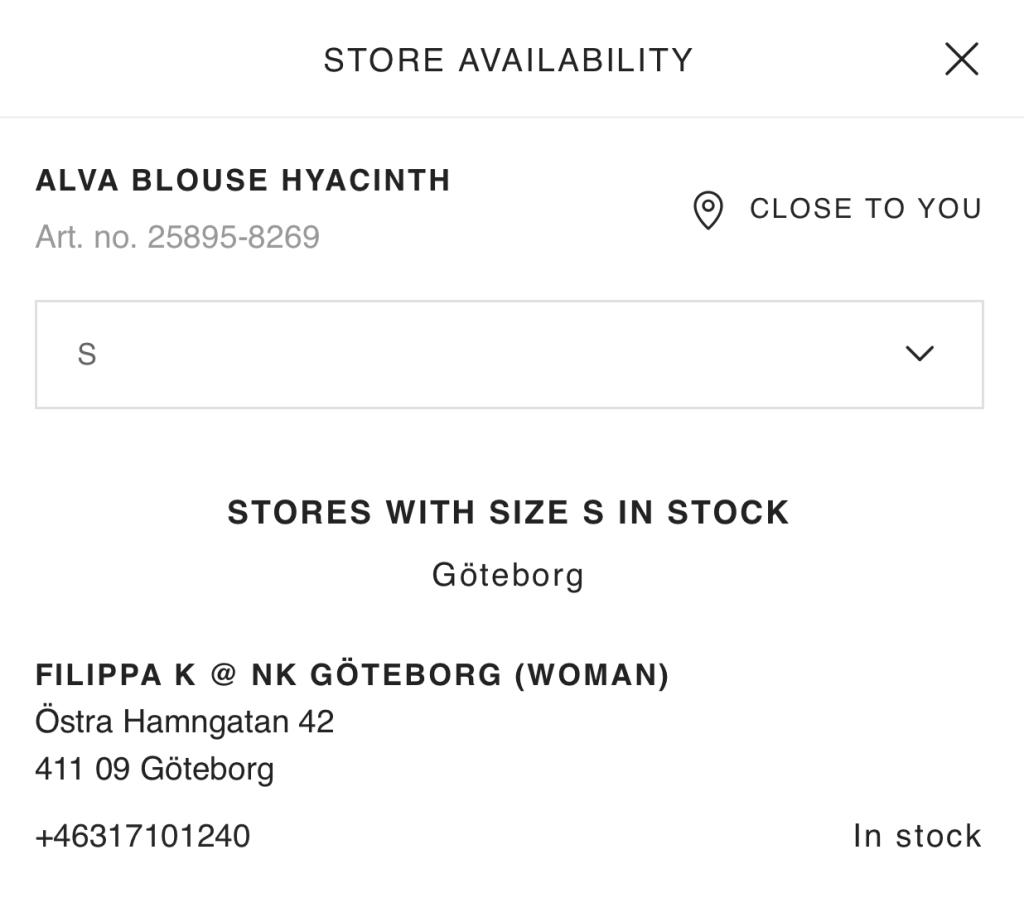
It allows a customer to check the availability not only by size, stores’ name and location, and also they can choose the store which is close to them. This allows for a customer to pick up the product in an offline store, and let you sell products to people that prefer to look at the product and try it on before they buy.
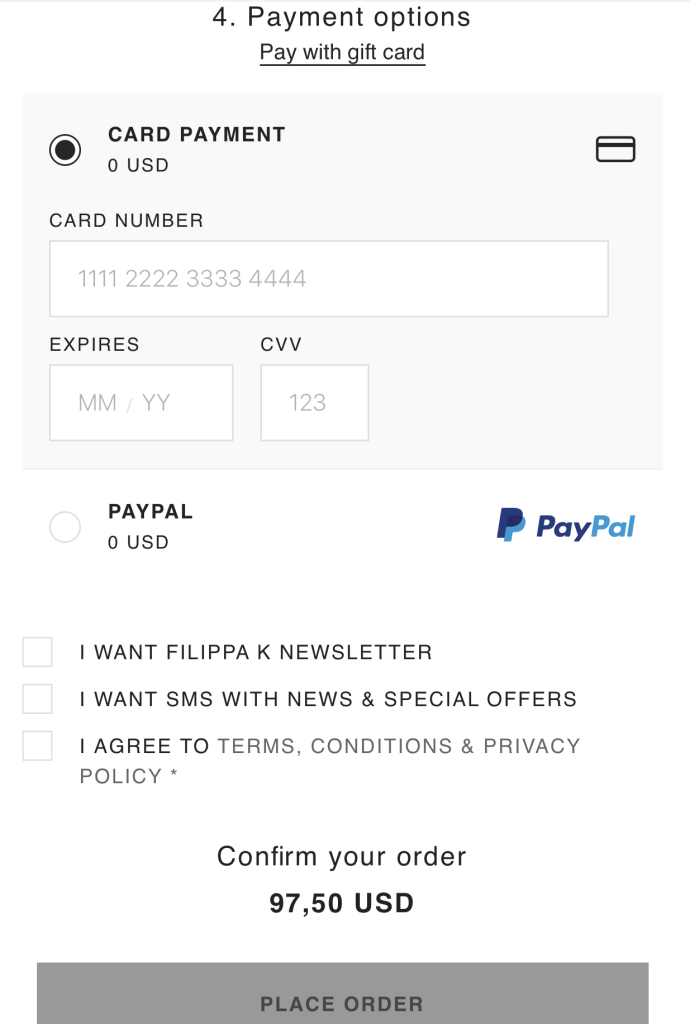
The mobile version also has one step checkout with 3 fields that you need to complete to place the order.
We’ve reviewed all the main features of the Filippa K online store. This strong brand continues to grow not only locally, but also on international markets sharing their mission of mindful consumption and timeless wardrobe. To achieve this goal, they use an online store which perfectly represents their vision, and helps their customers to buy products easily. If you want to grow your business, consider such functionality for your store.
Also, let’s sum up the most important insights from the review for your online business:
- Focus on your brand’s identity. Your brand is unique, and potential customers need to know about it. To give the right message, use your design, colors, vision, logo, and create a perfect design of your website. This will help your customers to distinguish you among others, and also strengthen your store positions on the market.
- Create an omnichannel experience. Connect both online and offline stores by adding necessary features such as availability of the product by location, detailed information about physical places, blog, or a separate page with social initiatives.
- Don’t forget about the cross-selling. You can sell more products by including related items or include others also bought feature to the checkout page. This provides customers with more options to purchase additional products that match their initial choice of items.
- Improve your product page look. Consider using an image slider, add a detailed product description, reviews, and a simple size guide, so your customers can learn everything about the product on one page. Let them feel confident about their choice, and become loyal admirers of your brand.
- Simplify the process of purchase. By adding a quick shop feature, simplify the order placement with one step checkout, you’ll save time for a customer to purchase products and lead them to order more from your store.
Working on the website look and feel isn’t an easy task, however, once your efforts will be rewarded with pleased and happy customers, you’ll be sure that everything that you’ve done before was worth it. We encourage you to showcase your brand in the best possible ways and let people know about the advantages of your store and its products.
Complete Guide to Finding the Best eCommerce Shipping Solutions
This article will help you to figure out what are the essential features for the eCommerce shipping software, provide with the list of top shipping solutions, and let you improve customer service and increase the number of completed orders.
Are you looking for new ways to ensure that your customers get the best shipping experience possible from your eCommerce store? If so, you’ve come to the right place. That’s because we have some essential tips to ensure that your customers can have a pleasant experience from shipping to delivery.
What is the eCommerce Shipping Solution?
An eCommerce shipping solution is a range of services from a shipping company which ensures that your customers receive their orders on time and intact. These services could carry out the questions of packing, labeling, shipping, delivery, and returns. Also, these shipping solutions often have analytics and reporting included in a plan, plus the ability to integrate POS solution to manage the delivery and warehouse stocks. Let’s look at the extensive list of features that a right shipping solution should have.
Useful for you:
Which Features Should It Include?
According to Narvar consumer report, 53% of online shoppers in the US won’t purchase a product if they don’t know its date of arrival. It means that online store customers expect clear information and care from the online store where they shop at the last stage of the buying process.
Shipping and delivery are crucial for customers according to different stats. Free shipping, accurate information, 24/7 support – all of this will ensure that a customer will complete their order. How to achieve this? By choosing the right eCommerce shipping software, you’ll save your time on managing the shipping process, and get more loyal clients. Below, you can learn about the features needed for the effective shipping solution.
Extensive List of Carriers
When deciding how to ensure that your customers receive their products safely, it is also vital that your shipping carrier is carefully vetted. The more carriers included in the shipping solution, the easiest way.
USPS
This shipping solution is a popular choice because it is common for shipping partners to offer eCommerce businesses discounts upon signing up with them. Among other advantages for your customers is the affordable price of their services compared to other shipping solutions because USPS does not charge for money when numerous shipping attempts are made.
FedEx and UPS
If you’re looking to ship a lot of heavier products, this may be the strain for you. That’s because they both accept shipments that weigh up to 150 pounds. These two shipping solutions also offer express delivery options.
DHL
This shipping solution ships around the world and offers good rates in the process. When you have packages shipped with DHL, you can have a discount of up to 66%.
Range of Shipping Methods and Rates
Different shipping methods will provide you with various benefits.
Free shipping is one of the best ways for you to get new customers and to keep old ones. This is because people, in general, are willing to pay more for products if they’re able to get free shipping. According to the recent survey of Efulfillment service, 74% of online shoppers prefer free shipping to complete the process checkout.
Another option for shipping is a flat rate. With this rate, you can charge a pre-set amount of money for every order. In doing so, your customers will know exactly how much to add for shipping at the end of their purchase.
You can, on the other hand, set a specific rate for different products. In doing so, you can edit prices to ensure that your shipping fee covers the rate of shipping it to a particular location.
Ability to Calculate Shipping Costs
You may want to include a feature that allows your eCommerce website to calculate the overall cost of shipping. Canada Post, USPS, and UK Royal Mail all have this feature; however, each shipping software always offers built-in calculator to perform custom calculations for the specific shipping carrier. As such, your customers can be aware of how much their shipping will cost before they continue with the purchase, and you’ll save your time in setting up shipping calculator additionally.
Tracking and Reporting Options
This is vital if you want to keep loyal customers who trust your services. When you use tracking and reporting options on your eCommerce website, you’re ensuring that your customers know where their package is at all times. This is a must feature for any shipping solution.
Build-In Analytics and Reporting
Built-in analytics provides you with real-time data that ensure you’re up-to-date with all information that is included in your shipping solution. This information will contain any late packages and any other relevant information that could come up.
Availability of Mobile App
It’s essential that you have a shipping solution that provides you with a mobile app. As such, you’re going to have an app that allows you to generate labels from anywhere, any time. While you may not be able to get the same functionality, a mobile app will let you to simplify the process of preparing items for shipping.
Shopping Cart Integration
Whichever shipping solution you choose, you should consider integrating it with your shopping cart. This will allow you to ensure that when your customers buy their products, a shipping solution is available to be updated and provide you with shipping data.
List of eCommerce Shipping Solutions
If you’re looking for an eCommerce shipping solution, you’re in luck. Below, you can read about the different options you have at your disposal.
ShipTheory
If you’re looking for a shipping method that can provide you with the best benefits, look no further. Here’s a full list of the pros and features.
- ShipTheory provides your business with a method to connect directly to a courier.
- With this tool, you can provide shipping to a wide number of individuals at a flexible and fair rate.
- You’ll be able to keep all of your shipping labels in the same place.
- The tool has several different packages you can benefit from. The prices range from being free to €300.
EasyShip
This shipping solution provides users with a number of benefits, all of which you can read below.
- EasyShip claims to help eCommerce business owners save up to 70%.
- You can choose from more than 250 different options and as such, can have your tracking information, labels, and more, all in one place.
- You can sell worldwide.
- The rates for EasyShip range from about $10 to $30, depending on your individual needs.
Landingo
Landingo can provide eCommerce users with shipping solutions, whether packages are small or large.
- Their main focus is on large packages.
- You can ship up to 150 pounds.
- You can track packages along the way.
- Paid service is included in the shipping price.
Shippit
Shipping provides eCommerce business owners with one vital thing: simplicity.
- With this tool, you can ensure that all of your shipping needs are entirely automated.
- An effective system of customers’ notification about the shipping process will decrease the number of possible issues.
- You can receive all of your data on a single platform.
- Prices range from about $20 to $150.
Easypost
If you’re looking for security in your solution, Easypost is the right one for you.
- Easypost provides eCommerce business owners with insurance, which makes sure that all packages are covered.
- With more than 100 carriers to their name, Easypost is a go-to solution for any package.
- You can pay as you go.
- This solution charges you 1 cent per package.
Shipstation
If you’re looking for a shipping solution for the Canadian market that focuses on the functionality you’ve come to the read place. Here is a shortlist of the benefits.
- Shipstation provides eCommerce business owners with a single place to create labels and ship packages.
- Shipments are updated in real-time to ensure that everyone is aware of every circumstance.
- Shipstation offers a free, one-month trial.
- Depending on how many shipments you need to have done every month, you can choose a package that ranges between $9 and $150.
Now you can choose among the top functional shipping solutions on the market. They will help you to expand your presence worldwide, manage a wide range of orders, and provide a qualitative shopping experience to your customers from the moment of order placement to the delivery of the items.
6 React.JS Advantages for Your Website
Before we dig into the benefits of this Javascript library, let’s start with history. A Facebook engineer, Jordan Walke, created React.js to simplify code maintenance and its updates for the Facebook Ads. Now, React.js is a flexible Javascript library. Because front-end development is always changing, it’s vital that eCommerce merchants stay up-to-date with the newest technologies and innovation.
Let’s see what are the benefits of React.js for website development.
Definition of React.JS
React.js, as previously mentioned, is known for being an open-source Javascript library that helps to build web applications without the need to reload pages. When it was first created, Facebook made it for use as an internal tool.
When to Use React?
React can be used whenever you have a complex web page or application to complete. It allows developing a project of any complexity which will be responsive, SEO-friendly, fast, and easy-to-upgrade.
Why Prefer ReactJS to Other Web Frameworks?
You might be wondering why I should choose to use React to get my website live? Well, the first thing is that you will get a great website that will have all the sophisticated features you need for your business. Also, there are some other benefits to take into account before choosing the framework for your site.
Why Creating Dynamic Sites Becomes Easier with React.js
One of the most attractive features of using React.js is that it can create more dynamic sites than the majority of its competition.
React Is Less Time-Consuming
Thanks to a flexible system, React allows developers to write clean and modular code, and breaking down the project into several components. It gives a clear look at the project and allows easily reuse the code after all.
This Javascript Library is Incredibly Versatile
React.js is one of the most desired frameworks because it is considered to be incredibly versatile. It allows developers to create clean code and manage updates. That’s because of all the components used in the development process are isolated from each other. As a result, changes can be made in any part and won’t affect the correct work of the website.
Because it is Open Source, you can also expect this library to provide you with more customization. From your general user interface to your shopping cart, you’ll be able to have a website that reflects your overall needs and priorities.
React is UI Responsive
A website’s user interface, otherwise known as UI, determines how users will interact with a website. React components for the design system, so-called Material UI help to get consistency across platforms, environments, and screen sizes giving you the responsive design.
A user interface that is responsive ensures that your visitors have a positive experience upon visiting your store. The faster your store is and the more efficient it is, the more likely your customers and visitors will return. With React, you’ll be able to get a responsive website and ensure that your customers will stay on your site’s pages longer and make expected actions.
It Has Seamless SEO Integration
Search engine optimization (SEO) is a method used to increase conversions and leads for websites. Things like keywords, meta descriptions, URLs, and more can be used to improve a website’s ranking in search engines like Google.
While search engines occasionally have difficult reading code that is Javascript-heavy, React has solved this by being easy to read by search engines. As such, you don’t need to worry about your ranking being negatively affected.
React Has a Big Community Around
React was originally created to serve internal systems and was then released to be used by developers and companies around the world.
According to SimilarTech, it is used by many companies – (currently 787,077 websites on React.js) and has a big community that continually improve this JS library and create additional modules for its better work.
It Has Support of Handy Tools
The tools that are used to develop your site ensure that your site is customized to the best of its ability and that it suits your individual needs. Tools such as Bit, StoryBook, Reactide, ReactStudio, and others help to improve the design, collect all the components for the development, render pages quickly, and ensure that the whole development process is performed in a simpler way. This allows to simplify the development process and build better websites.
Stutterheim Store Review
Here is another review of the successful clothing store with a creative background. With Stutterheim’s example, you’ll see how one small idea can grow into a strong and successful brand. We’ll explore their website and highlight the best features that help to get its act together and attract more customers.
Stutterheim is a Swedish company with genuine creativity on melancholy and perfect raincoats. As well as their high-quality rain clothes, the brand has a rich background.
Stutterheim’s story began with the fisherman’s coat that was found on a rainy day. The creative director, Alexander Stutterheim, found his grandfather’s old raincoat, and he noticed that Swedes didn’t have any proper clothing for rainy days. So, he decided to redesign that raincoat into a modern version. That’s where the successful journey of this brand began.
Working on new raincoats, Alexander Stutterheim and his friend, marketing director, and co-founder, Johan Loman, found a unique meaning of melancholy, and it has fundamentally changed the relation to this term. Now, the story about the grandfather’s coat, melancholy, and creativity is combined into a strong brand of Swedish raincoats.
After you realize the brand’s story, concept, and values, you will find out how they develop the store. This guide will help you to choose the useful features for your online clothing store and improve user-experience by following the example of the Stutterheim brand. We also want to share our latest interview with Stutterheim’s CEO, Peter Bergkrantz on brand growth and development online, which is a great addition to the article. This will give you the full picture of the brand, their values, and why it is successful on the market.
We’ll begin with a desktop site version and then continue with the website appearance on the mobile version. This will guide you through the idea of how the company manages their online store. Let’s move on to the review.
Company’s Site Overview
When you first enter the home page, perhaps, you expect to see some dark colors, strict design, and a rainy mood. Instead, you get a colorful banner that tells you about the summer sale and connects you with the colors of the latest collections. Below you get shipping information, bestsellers pieces, and some main store categories, which make it easy for a customer to go to the product listing.
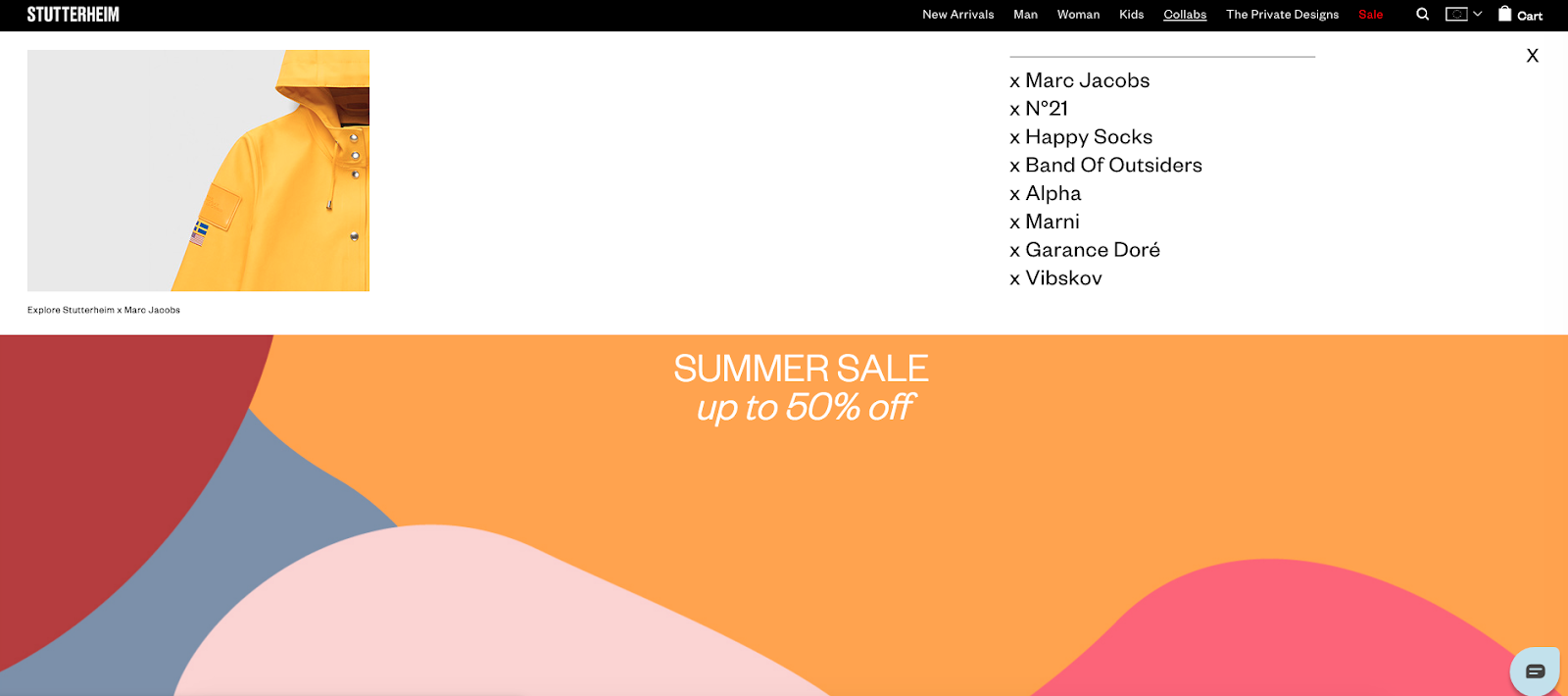
The design is very stylish and follows the melancholic philosophy of the brand. If you click on the multistore selection, you see the flags in black&white colors, which is pretty cool and again follows the brand’s style.

Functionality Analysis
The website has a common store features as:
We’ve started with a short list of the main Stutterheim features. Let’s move on to the detailed overview and consider which functionality is essential for online clothing store on desktop and mobile.
Desktop
Home Page & Navigation
When you enter the main page, first, you see that the store has main categories and some featured collections named Collabs and Private Designs. Except for their iconic Arholma coat, customers can now choose shoes, hats, thermal liners, knitwear, and accessories.
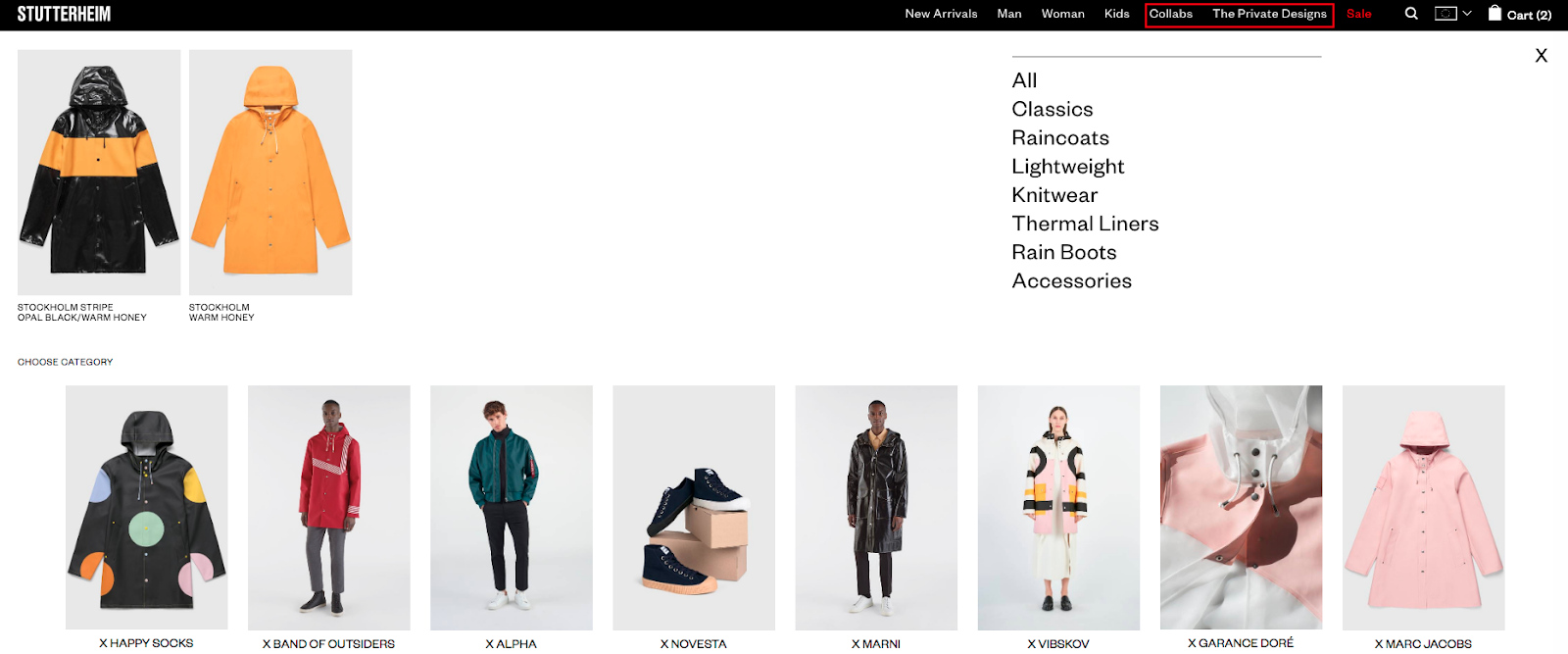
We mentioned featured sections because it is a great way to present your customers with some custom products, make them feel unique. Increase brand awareness through cooperation with famous companies, influencers, and other famous people when creating new collections. This will also lead your customers to get more items from your store and overall sales increase.
Among other features presented on the main page is the Instagram block.
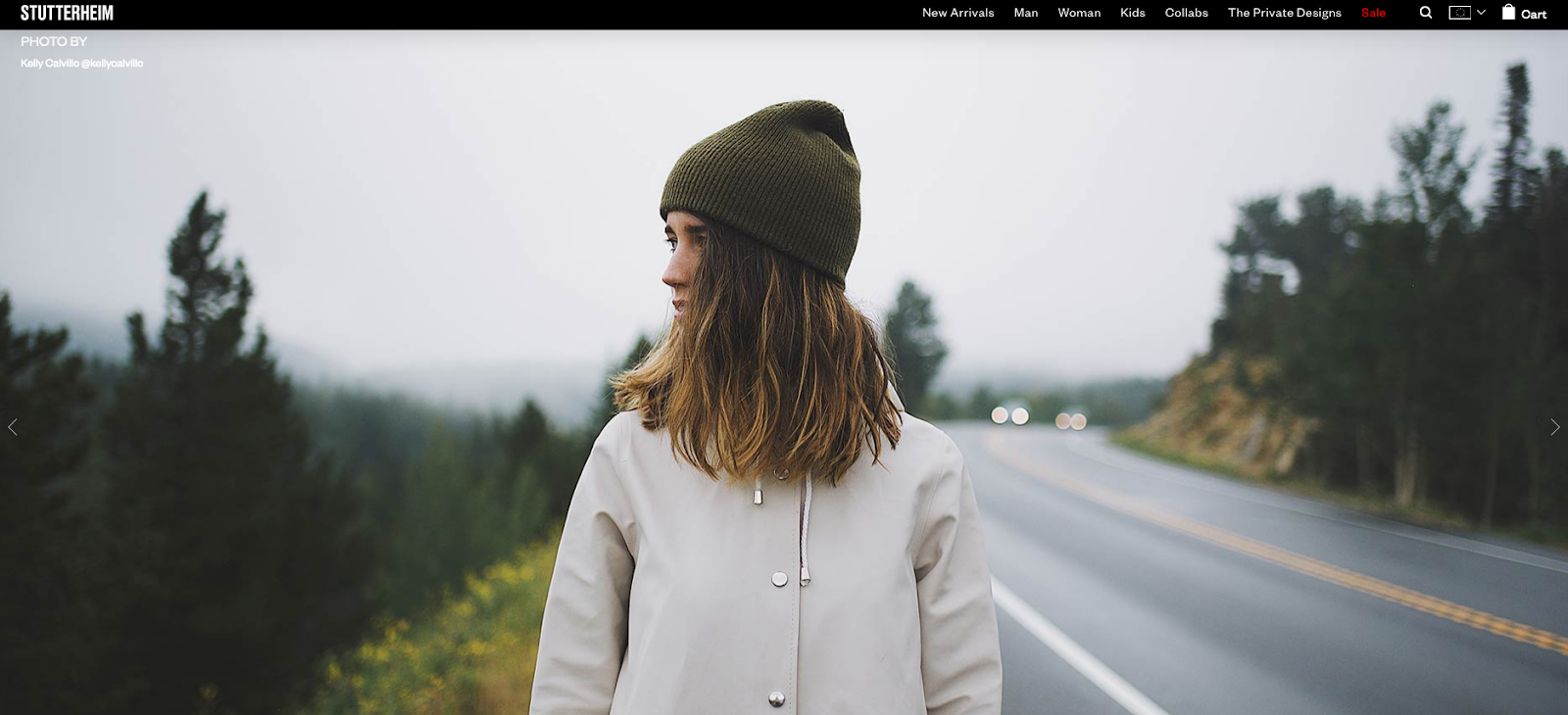
If you click on a picture, you go to the #Stutterheim page, where you’ll see Instagram pictures with customers wearing Stutterheim clothing. The process is simple: a customer posts the Instagram picture and adds a hashtag featuring Stutterheim, and then it automatically appears on the main page of the website. It is a useful add-on that lets a customer get the desired look in a single click. Also, it is an excellent channel for promotion, and it gives an overview of how the product looks on a real person.
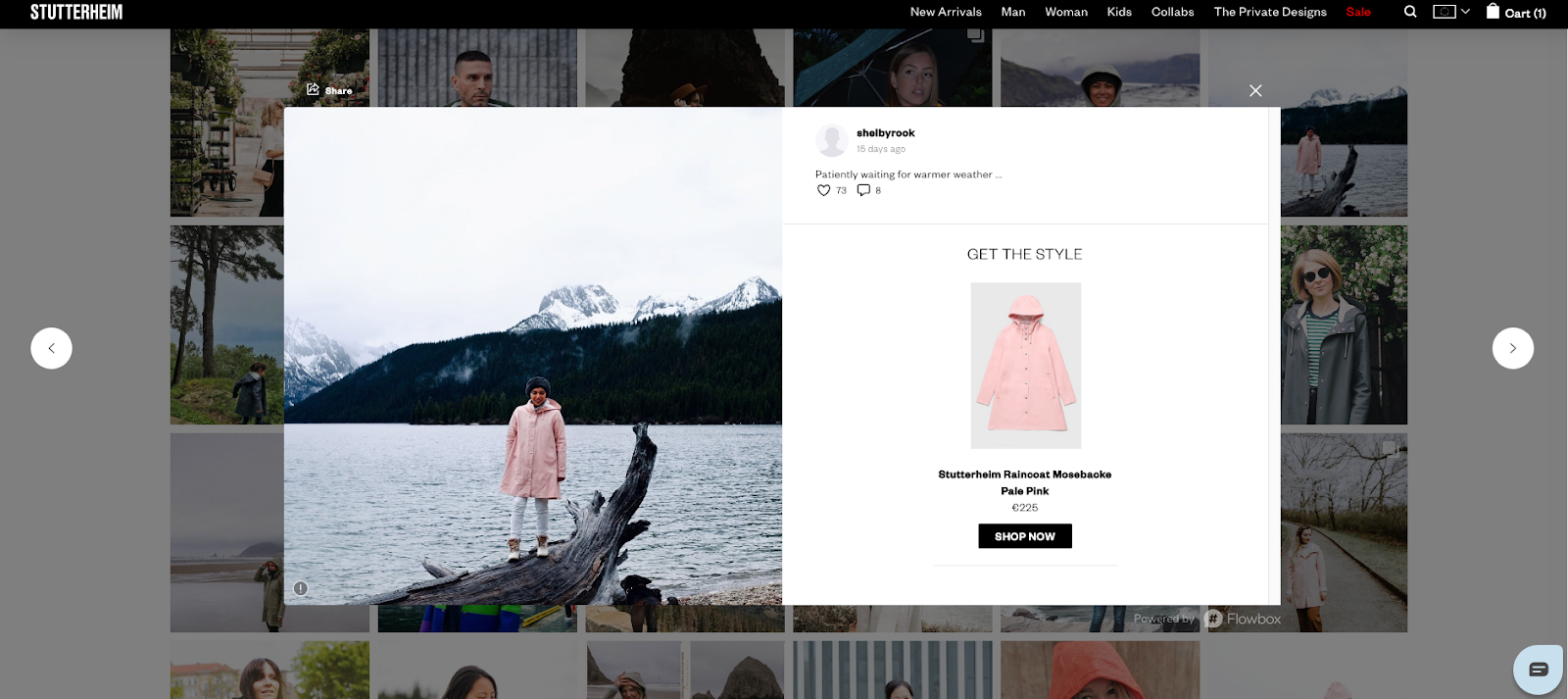
Also, you can find the same block on the bottom of the product page. Customers can look at the product worn by a real person.
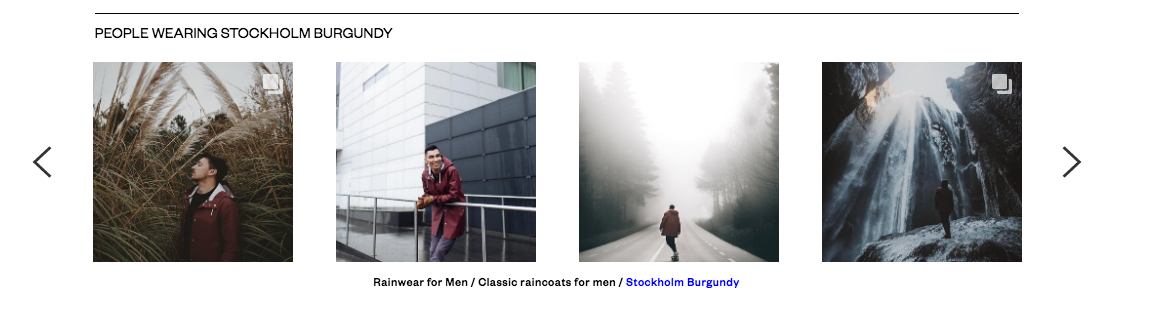
Product and Category Pages
Each category has simple navigation by sections, as well as a filter with a small range of options. Filters are accessible, and you can choose any parameter from the 1st section, which doesn’t distract a user from the product search.
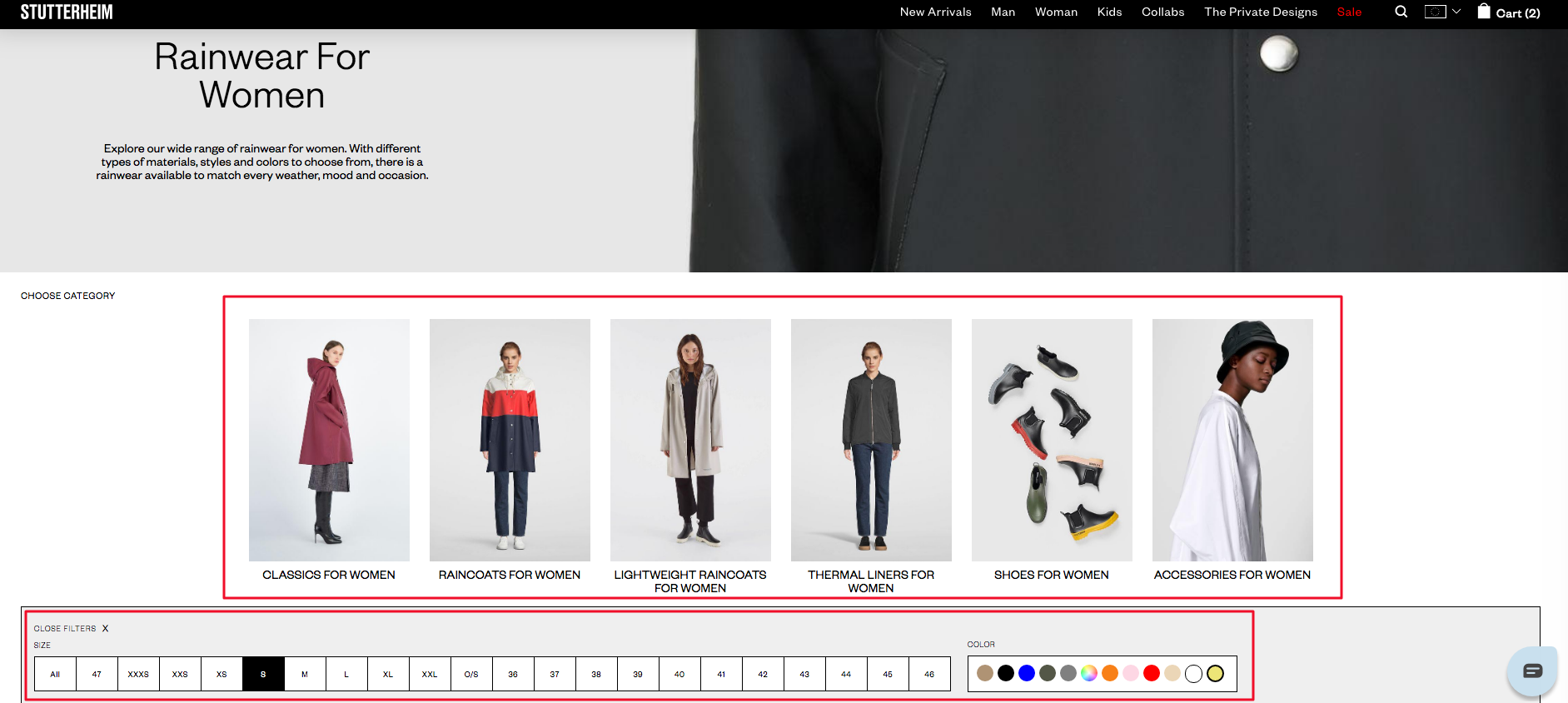
After a user chooses the product from listing, they go to the product page. Each product page has Images, Size Chart, Size Guide (with Virtual Fit Guide), Add to Cart button, Description, Shipping, and Returns, You May Also Like, and People Wear.
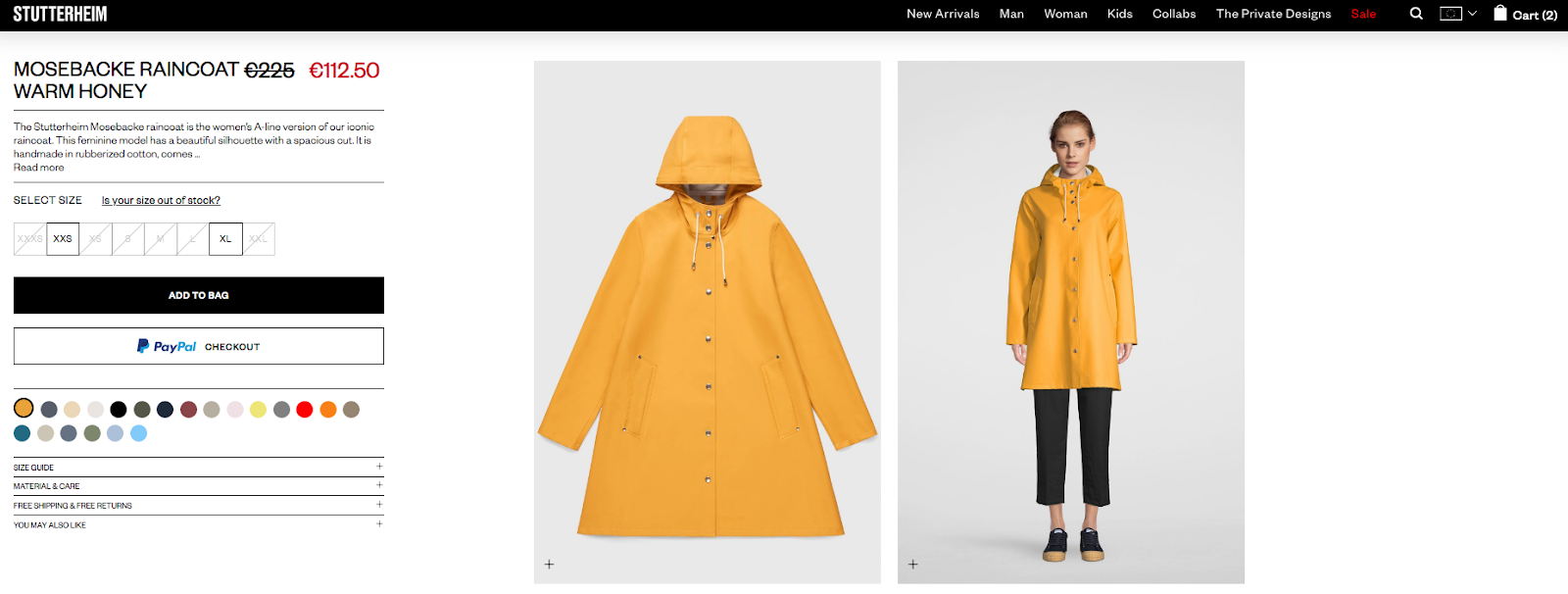
Sections presented in a dropdown menu save space on the page. Resizing the pictures helps a customer to look at the product from different angles. As for the size selection, it is performed in a simple way allowing a customer to see which sizes are available. Particular sizes of items that are out-of-stock are crossed and highlighted with a lighter color, which helps a customer to see only available sizes of the specific product.
If we look at the special features on this page, definitely PayPal Checkout and Virtual Fit Guide elevate the shopping experience to a high level.
PayPal Smart Payment Button allows a customer to pay orders through the PayPal account and place it in one click. This feature cuts off the time for the order placement and lets a customer save their time.
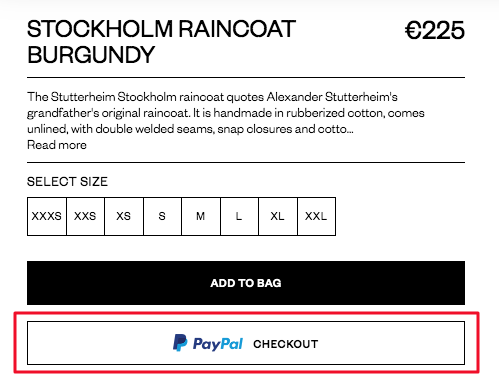
Virtual Fit Guide allows the customer to check how the clothing fits them based on their parameters. You write width, height, and other data, then the tool shows you the store clothing parameters and compares it to yours, giving some general recommendations regarding your choice.
Checkout Page
The checkout page needs to be easy to use. In Stutterheim’s case, the checkout opens on the same page so that the user can see their cart immediately. This is one of the most popular types of checkout, and it doesn’t distract a user from the purchase.
Let’s see how it works. A customer adds some products to the cart. After that, they get a side pop-up with items added and can proceed to checkout.
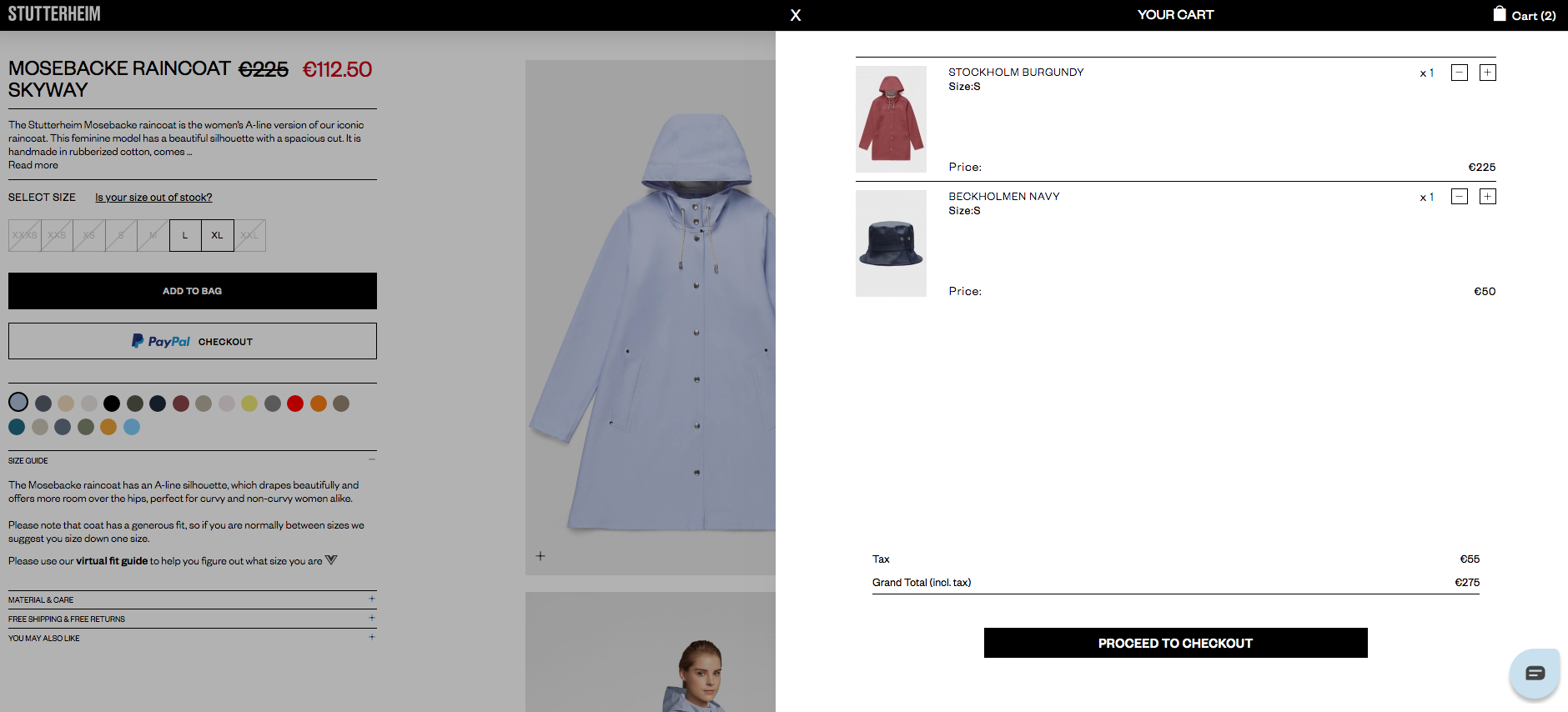
If someone chooses to proceed to the checkout instead of fast PayPal Checkout, they need to fill out the contact information and then pay with a credit card or PayPal once again. Then a customer places the order and waits for the email with details.
Marketing Pages
The informational part of the site includes a Blog, an Instagram block, and an Our Story page. They help to involve a customer into the history of the brand, allowing more touchpoints while browsing the store.
One of the best ways to present the brand’s uniqueness is to create some story or associate your brand with some concept to have a strong position in people’s minds. That’s why Stutterheim decided to connect the brand with a Melancholy term. Instead of its original meaning, they made melancholy a part of their creative strategy and highlighted talented people talking about melancholy and art in small interviews.
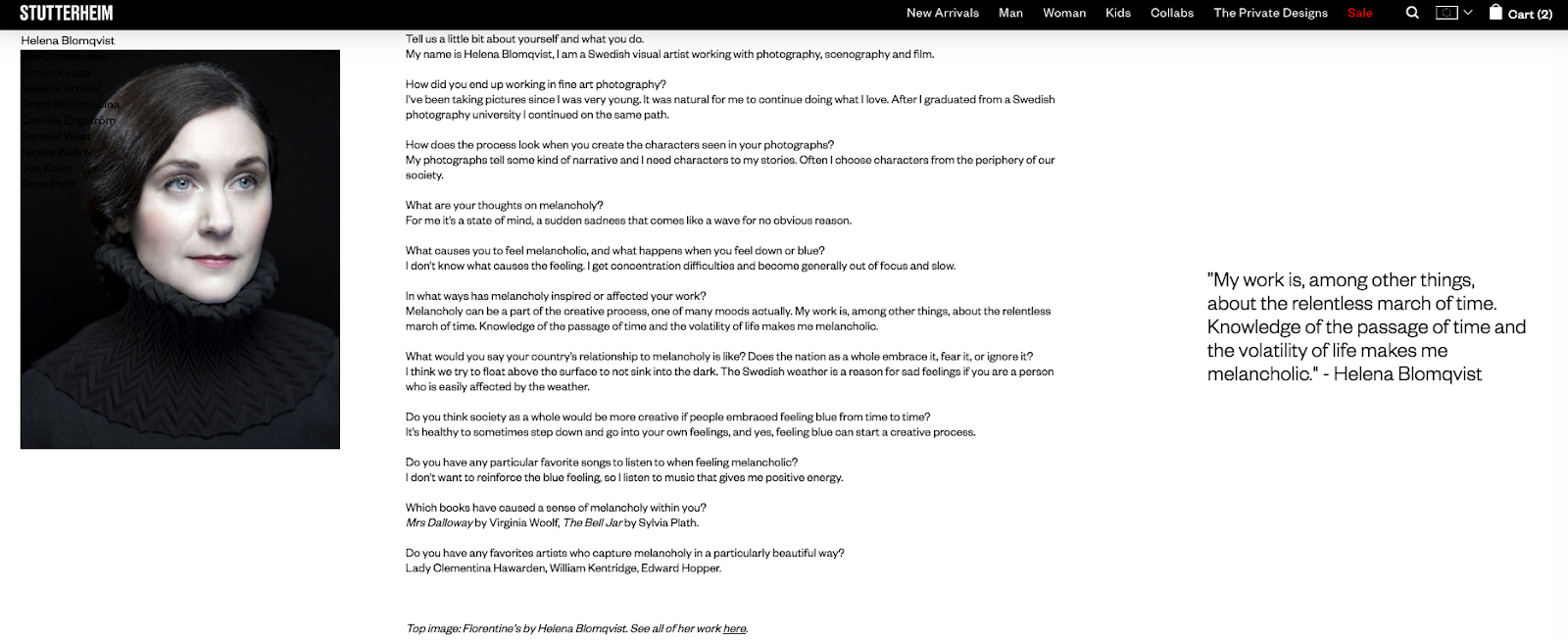
If the brand features their background and highlights the key dates on one page, it will work for the increase of brand awareness. That’s what Stutterheim did in presenting their history with a detailed description of each stage.
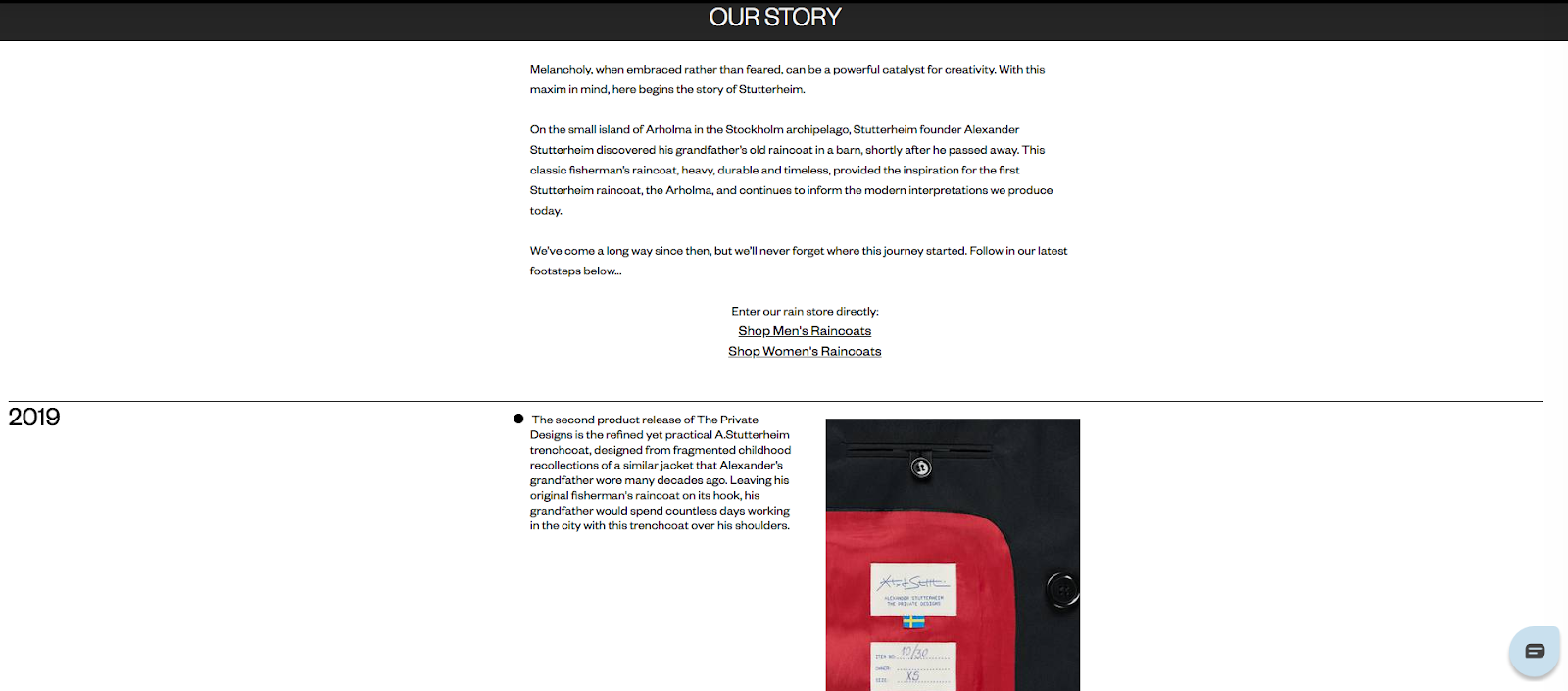
As far as we can see, the desktop version of the website is convenient for the website user and helps to communicate brand vision and values.
What about mobile visitors?
Do you know that mobile devices make up about 23% of overall eCommerce website traffic?
What about the Stutterheim stats? According to Semrush, mobile traffic consists of 37% of the total traffic, which mostly comes from the United States. Scandinavian users visit the website only using mobile devices.
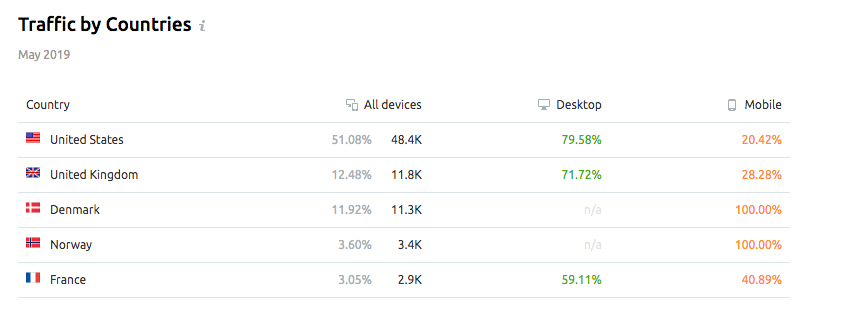
Let’s move on to the Mobile site version and see how well the Stutterheim brand takes care of their mobile visitors and what helps them to convert their website visitors into loyal customers.
Mobile
The mobile version is well-optimized and easy-to-browse. The main page repeats the desktop version. The mobile hamburger menu guides you to the categories, which is convenient and easy to click on the smartphone.
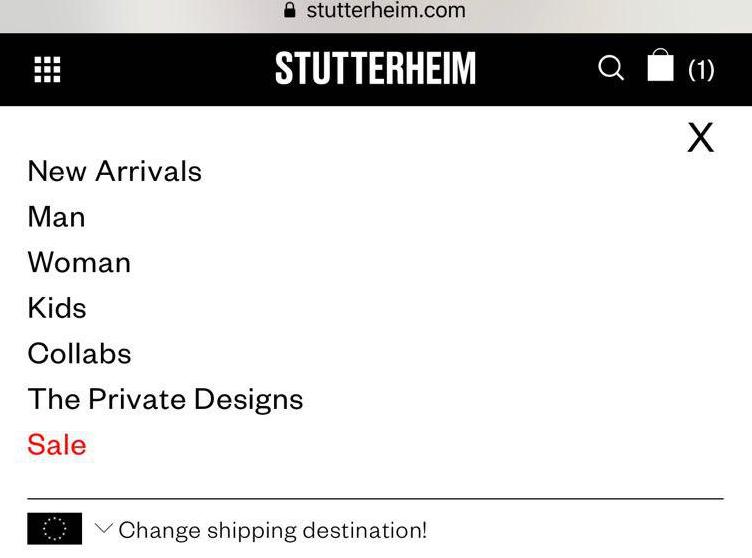
Below in footer, there is a dropdown menu with all site categories. It is convenient in case you have lots of essential pages that you want to highlight.
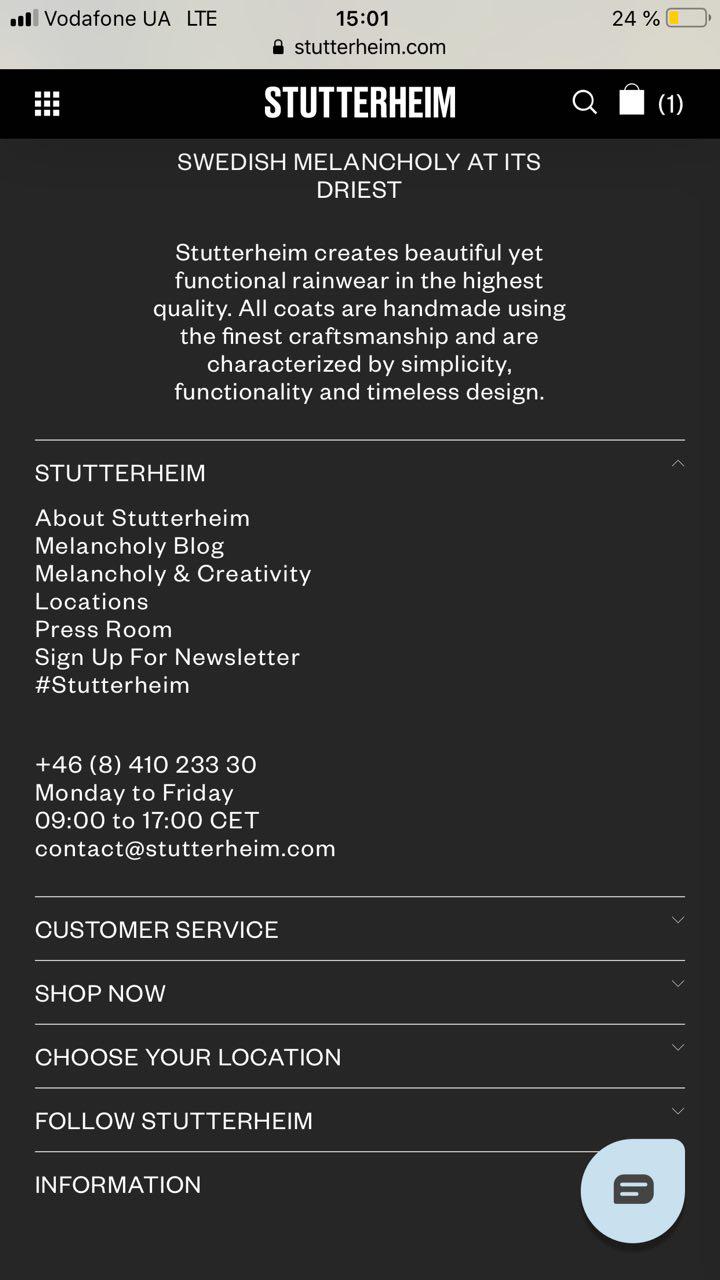
Category and Product pages are also simple to browse, which makes the mobile version completely user-friendly.
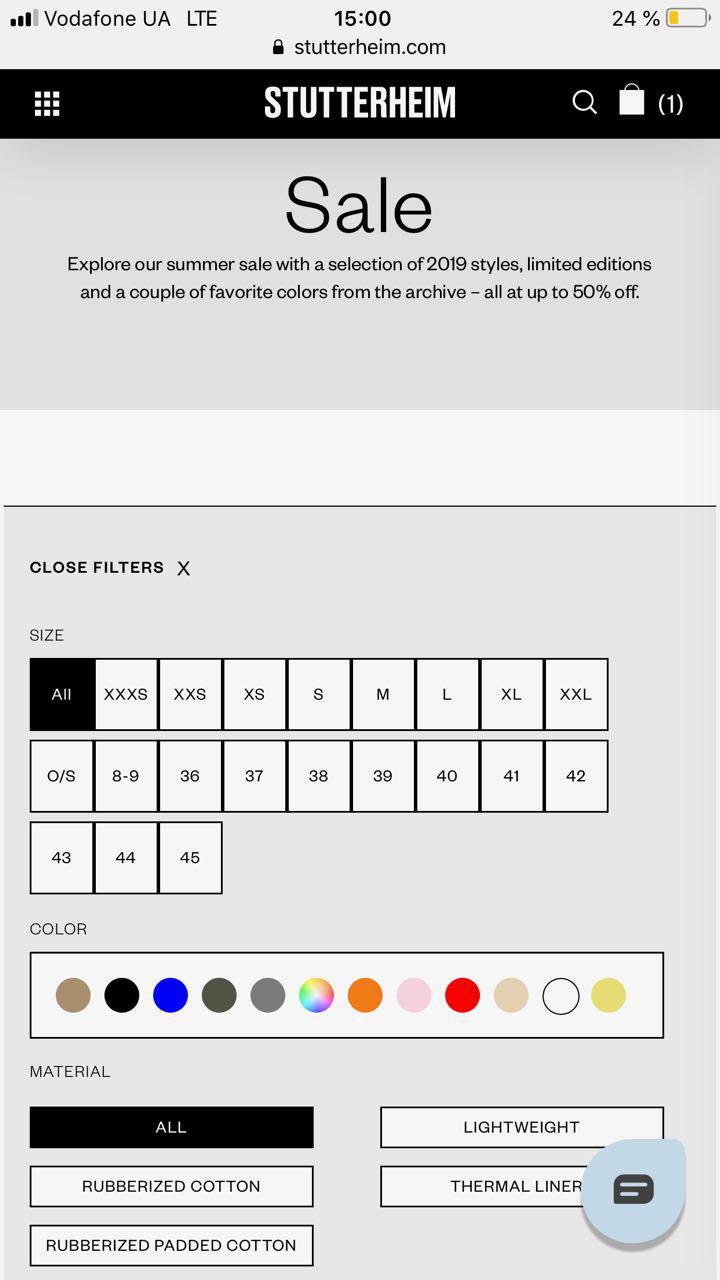
You can apply any filters by size, color, and material. The product page also has all the descriptions, Size Guide, Add to Cart, Virtual Size app, Swatches, PayPal Checkout, and Shipping information. It is easy-to-click on each line, and overall, the information is accessible.
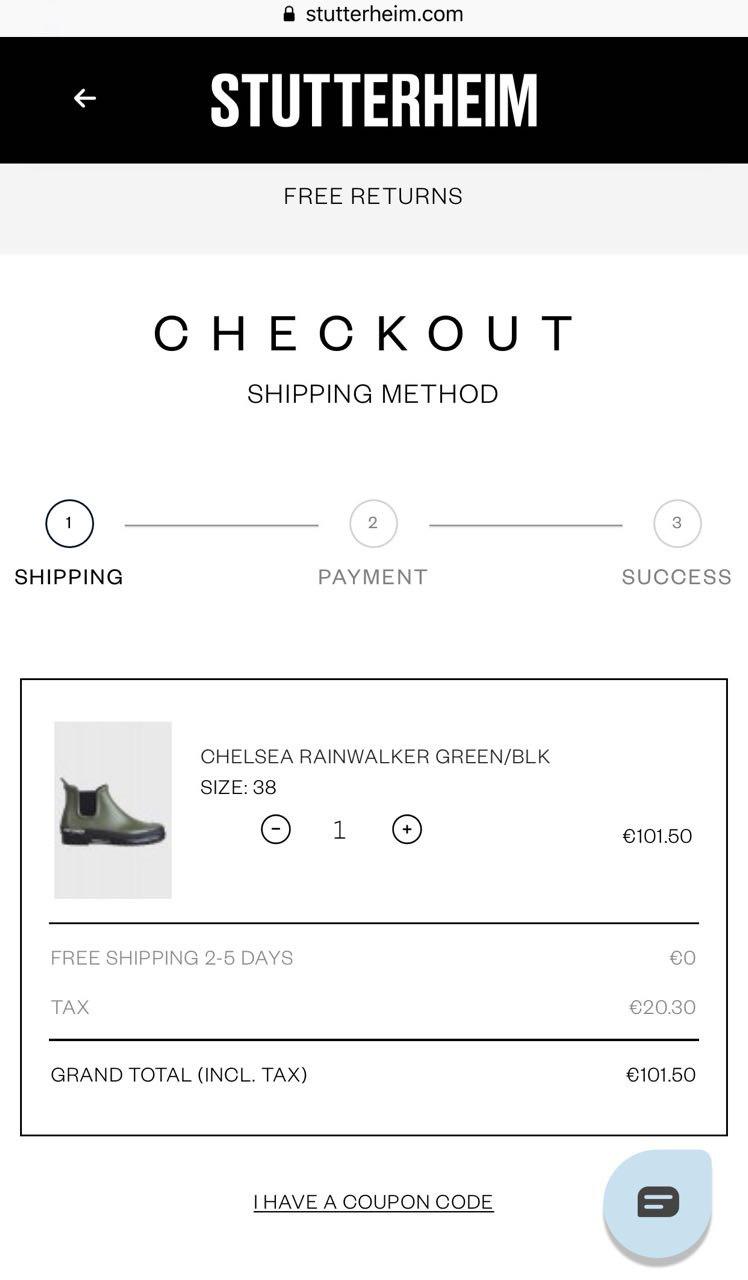
The mobile version also has a two-step checkout and splits total to a tax and a total sum. Also, the store offers free shipping and returns, which is appreciated by customers. Such a simple checkout with price highlights lets a customer be aware of their total payment. That makes them confident about their purchase, and let them return to your store again.
So, we’ve considered the main aspects of the online store. Stutterheim has created great products for rainy days, and fully functional website that makes a shopping experience a pleasant thing. If you wanted to find a great example for your future store, consider such features and structure from this brand’s website.
Here we want to place the main highlights and tips from this review for your business:
- Communicate your brand’s positioning. Tell the story of your brand using creative storytelling and highlight your exception on the About us page. Communicate your brand’s value through your mission, goals, and visual design. Be honest, sincere, and authentic to have a place in people’s minds.
- Use the power of social media. Highlight your customers’ publications via their Instagram on a separate page. Let others see how great and accessible your products are, and how they look on real people. Involve some influencers, and other brands, and create new collections with them to reach a wider audience.
- Make clear website navigation. To simplify your site navigation, you can start with making a clear menu with minimum categories, including important pages, and optimize filters with relevant parts such as size, color, material, and product type. Overall think about every step of the customer’s interaction with your store, which means the accessibility of pages for a customer.
- Improve your site search. Besides a regular search that should be included in all website pages, you can upgrade it with a plugin that allows you to have autosuggestions and autocomplete, which will simplify the search and save time for a customer to find the exact product.
- Increase the accessibility of each page. Information related to the product, payment, shipping, and delivery is essential if you want your customers to complete their purchase and come back to your store again and again. All the necessary pages should be visible and reached in two or three clicks. You can use the sidebar for each page to help customers reach them quickly.
- Realize your business goals with the store’s features. Include CTA buttons below the product. Place all the current offerings on main banners, and the menu so the customer can check them quickly. This also includes each feature that will help you to reach your customers, such as sending newsletters and reminders for the abandoned carts, related products, and you may also like block and simple search.
- Simplify search of the size. Implement Virtusize fitting solution into your size guide, and help customers to define their size easier.
- Provide various payment options. Use PayPal Checkout to simplify order placement for your customers. Also, don’t forget about the security level.
- Let your customers feel secure and valuable. Add live chat, and reCAPTCHA, so customers will see that the store is safe, and the team is supportive. Also, include the store locator, detailed description of each office, and store. This helps to connect customers with local stores and allow them to buy offline, which means improvement of the omnichannel experience
- Optimize the buying process. Cart and checkout should have clickable products, the ability to add any product quantity, plain fill out the form with autocomplete, and order confirmation as a pop-up, and email notification. When a customer adds any product to cart, you may include the feature Others also buy on the product page or at the cart page, so the customer gets a chance to buy more products, and you’ll get more sales.
Now you can use some insights and features for your store to elevate it to the new level.
The fashion industry is growing; the competitive market is also developing. However, any brand with a great idea and story has their opportunity to gain the trust and love of their customers and go skyrocket. You can build your brand and take care of the convenience of your website visitors; improve the user experience at your store, and let your business continuously grow and strengthen positions on the market, as the Stutterheim did.
Upgrading Magento to 2.2.8 While Using Mageplaza Smtp Extension 1.2.9: Fixing Errors
The Magento 2 era began on November 17, 2015, when Magento released the first version of its 2.0 line. Since then, Magento has been launching updates one after another, while sometimes supporting several branches at the same time. Magento 2.0 is no longer supported, but subsequent versions (2.1, 2.2, 2.3) still are. Magento supports several branches simultaneously because different systems have different requirements. For example, Magento 2.1 still supports PHP 5.6, whereas the minimum version of PHP for Magento 2.2 is 7.0.13 and for Magento 2.3 it’s 7.1.3.
It’s not always feasible for store owners to update to new versions. Moreover, stores usually contain third-party modules which, broadly speaking, shouldn’t conflict with new versions of Magento, although in reality they sometimes do. So even though Magento 2.3 was released on November 28, 2018, many store owners are still using version 2.2 and will be for quite a while.
On March 26, 2019, Magento released the three latest versions in each branch: 2.1.7, 2.2.8, and 2.3.1. We would like to discuss working with the extension for version 2.2.8.
Background on the Mageplaza Smtp Extension
Third-party extensions are often used for sending emails. One of these extensions is the module Mageplaza Smtp. This extension is free, which is why it’s popular. That being said, after upgrading the core to version 2.2.8 and the Mageplaza module to version 1.2.9, the site stopped sending emails. We will investigate why this happened.
Let’s analyze the Mageplaza Smtp module. It is embedded in Magento for sending emails, using the following plugin:
<type name="Magento\Framework\Mail\TransportInterface">
<plugin name="mageplaza_mail_transport" type="Mageplaza\Smtp\Mail\Transport" sortOrder="1" disabled="false"/>
</type>
We are interested in the constructor and only one method of this plugin:
public function __construct(
Mail $resourceMail,
LogFactory $logFactory,
Registry $registry,
Data $helper,
LoggerInterface $logger
)
{
$this->resourceMail = $resourceMail;
$this->logFactory = $logFactory;
$this->registry = $registry;
$this->helper = $helper;
$this->logger = $logger;
}
/**
* @param TransportInterface $subject
* @param \Closure $proceed
* @throws MailException
* @throws \Zend_Exception
*/
public function aroundSendMessage(
TransportInterface $subject,
\Closure $proceed
)
{
$this->_storeId = $this->registry->registry('mp_smtp_store_id');
$message = $this->getMessage($subject);
if ($this->resourceMail->isModuleEnable($this->_storeId) && $message) {
if ($this->helper->versionCompare('2.3.0')) {
$message = \Zend\Mail\Message::fromString($message->getRawMessage());
}
$message = $this->resourceMail->processMessage($message, $this->_storeId);
$transport = $this->resourceMail->getTransport($this->_storeId);
try {
if (!$this->resourceMail->isDeveloperMode($this->_storeId)) {
$transport->send($message);
}
$this->emailLog($message);
} catch (\Exception $e) {
$this->emailLog($message, false);
throw new MailException(new Phrase($e->getMessage()), $e);
}
} else {
$proceed();
}
}
As we can see, it checks the Magento version and executes additional code if the version is greater than or equal to 2.3.0.Why does this happen? Magento’s main library is Zend Framework. However, for historical reasons, Magento 2 contains both Zend Framework 1 classes and Zend Framework 2 classes. For a long time, Magento used Zend Framework 1 classes to send emails, but there was a transition to Zend Framework 2 classes in version 2.3.0. The use of Zend Framework 2 libraries were then added to version 2.2.8 as well.
It’s likely that the Mageplaza developer team didn’t know this when they released this version, so they created a check for version 2.3.0, which made the Mageplaza Smtp 1.2.9 extension incompatible with Magento 2.2.8. That being said, this conflict can be resolved.
Solution for Compatibility Between Version 1.2.9 of the Mageplaza Smtp Module and Magento 2.2.8
Let’s look at which other classes are checked for the version. These classes are Mageplaza\Smtp\Mail\Rse\Mail and Mageplaza\Smtp\Model\Log. In these classes, there is a check for version 2.3.0. Let’s rework the comparison to check for 2.2.8. The actual solution to this problem looks as follows:
di.xml
<type name="Magentice\All\Model\Mail\Transport">
<arguments>
<argument name="resourceMail" xsi:type="object">Web4pro\All\Model\Mail</argument>
</arguments>
</type>
<type name="Mageplaza\Smtp\Plugin\Message">
<arguments>
<argument name="resourceMail" xsi:type="object">Web4pro\All\Model\Mail</argument>
</arguments>
</type>
<preference for="Mageplaza\Smtp\Mail\Transport" type="Web4pro\All\Model\Mail\Transport"/>
<type name="Mageplaza\Smtp\Model\Log">
<plugin name="fix-mageplaz-228-incompability" type="Web4pro\All\Model\Plugin" sortOrder="20"/>
</type>
The classes will look as follows:
Web4pro\All\Model\Mail\Transport
class Transport extends \Mageplaza\Smtp\Mail\Transport
{
public function aroundSendMessage(
TransportInterface $subject,
\Closure $proceed
)
{
$this->_storeId = $this->registry->registry('mp_smtp_store_id');
$message = $this->getMessage($subject);
if ($this->resourceMail->isModuleEnable($this->_storeId) && $message) {
if ($this->helper->versionCompare('2.2.8')) {
$message = \Zend\Mail\Message::fromString($message->getRawMessage());
}
$message = $this->resourceMail->processMessage($message, $this->_storeId);
$transport = $this->resourceMail->getTransport($this->_storeId);
try {
if (!$this->resourceMail->isDeveloperMode($this->_storeId)) {
$transport->send($message);
}
$this->emailLog($message);
} catch (\Exception $e) {
$this->emailLog($message, false);
throw new MailException(new Phrase($e->getMessage()), $e);
}
} else {
$proceed();
}
}
}
This class redefines the around plugin from Mageplaza_Smtp.
Web4pro\All\Model\Mail
class Mail extends \Mageplaza\Smtp\Mail\Rse\Mail
{
public function processMessage($message, $storeId)
{
if (!isset($this->_returnPath[$storeId])) {
$this->_returnPath[$storeId] = $this->smtpHelper->getSmtpConfig('return_path_email', $storeId);
}
if ($this->_returnPath[$storeId]) {
if ($this->smtpHelper->versionCompare('2.3.0')) {
$message->getHeaders()->addHeaders(["Return-Path" => $this->_returnPath[$storeId]]);
} else if (method_exists($message, 'setReturnPath')) {
$message->setReturnPath($this->_returnPath[$storeId]);
}
}
if (!empty($this->_fromByStore) &&
(($message instanceof \Zend\Mail\Message && !$message->getFrom()->count()))
) {
$message->setFrom($this->_fromByStore['email'], $this->_fromByStore['name']);
}
return $message;
}
public function getTransport($storeId)
{
if (null === $this->_transport) {
if (!isset($this->_smtpOptions[$storeId])) {
$configData = $this->smtpHelper->getSmtpConfig('', $storeId);
$options = [
'host' => isset($configData['host']) ? $configData['host'] : '',
'port' => isset($configData['port']) ? $configData['port'] : ''
];
if (isset($configData['authentication']) && $configData['authentication'] !== "") {
$options = [
'auth' => $configData['authentication'],
'username' => isset($configData['username']) ? $configData['username'] : '',
'password' => $this->smtpHelper->getPassword($storeId)
];
}
if (isset($configData['protocol']) && $configData['protocol'] !== "") {
$options['ssl'] = $configData['protocol'];
}
$this->_smtpOptions[$storeId] = $options;
}
if (!isset($this->_smtpOptions[$storeId]['host']) || !$this->_smtpOptions[$storeId]['host']) {
throw new \Zend_Exception('A host is necessary for smtp transport, but none was given');
}
if ($this->smtpHelper->versionCompare('2.2.8')) {
$options = $this->_smtpOptions[$storeId];
if (isset($options['auth'])) {
$options['connection_class'] = $options['auth'];
$options['connection_config'] = [
'username' => $options['username'],
'password' => $options['password']
];
unset($options['auth']);
unset($options['username']);
unset($options['password']);
}
if (isset($options['ssl'])) {
$options['connection_config']['ssl'] = $options['ssl'];
unset($options['ssl']);
}
unset($options['type']);
$options = new \Zend\Mail\Transport\SmtpOptions($options);
$this->_transport = new \Zend\Mail\Transport\Smtp($options);
} else {
$this->_transport = new \Zend_Mail_Transport_Smtp($this->_smtpOptions[$storeId]['host'], $this->_smtpOptions[$storeId]);
}
}
return $this->_transport;
}
Web4pro\All\Model\Plugin
class Plugin
{
protected $productMetaData;
public function __construct(\Magento\Framework\App\ProductMetadata $productMetaData)
{
$this->productMetaData = $productMetaData;
}
public function aroundSaveLog($subject,$proceed,$message,$status){
if(version_compare($this->productMetaData->getVersion(),'2.2.8','<')){
$proceed($message,$status);
}else{
if ($message->getSubject()) {
$subject->setSubject($message->getSubject());
}
$from = $message->getFrom();
if (count($from)) {
$from->rewind();
$subject->setSender($from->current()->getEmail());
}
$toArr = [];
foreach($message->getTo() as $toAddr){
$toArr[] = $toAddr->getEmail();
}
$subject->setRecipient(implode(',', $toArr));
$ccArr = [];
foreach($message->getCc() as $ccAddr){
$ccArr[] = $ccAddr->getEmail();
}
$subject->setCc(implode(',', $ccArr));
$bccArr = [];
foreach($message->getBcc() as $bccAddr){
$bccArr[] = $bccAddr->getEmail();
}
$subject->setBcc(implode(',', $bccArr));
$content = htmlspecialchars($message->getBodyText());
$subject->setEmailContent($content)
->setStatus($status)
->save();
}
}